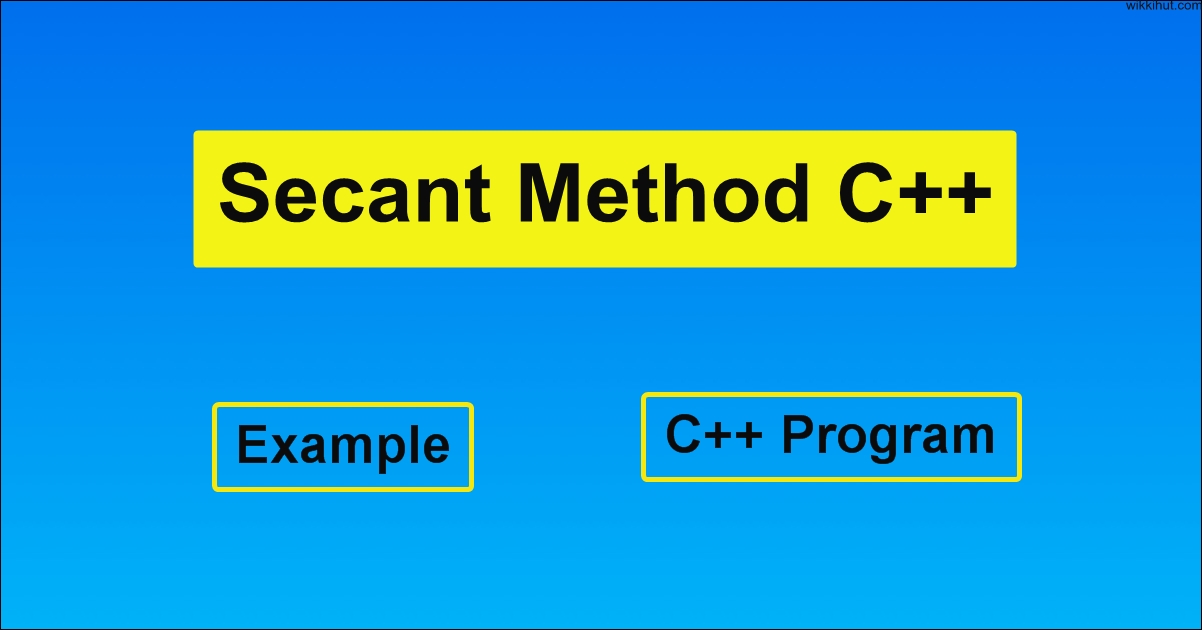
What is Secant Method
Intro:
The Secant Method is used to find the roots of an equation.
The Secant method is similar to the Regula-Falsi method, except for the fact that we drop the condition that f(x) should have opposite signs at the two points used to generate the next approximation.
Instead, we always retain the last two points to generate the next. Thus, if xi-1 and xi are two approximations to the root, then the next approximation xi+1 to root is given by the following equation.
Secant Method Formula
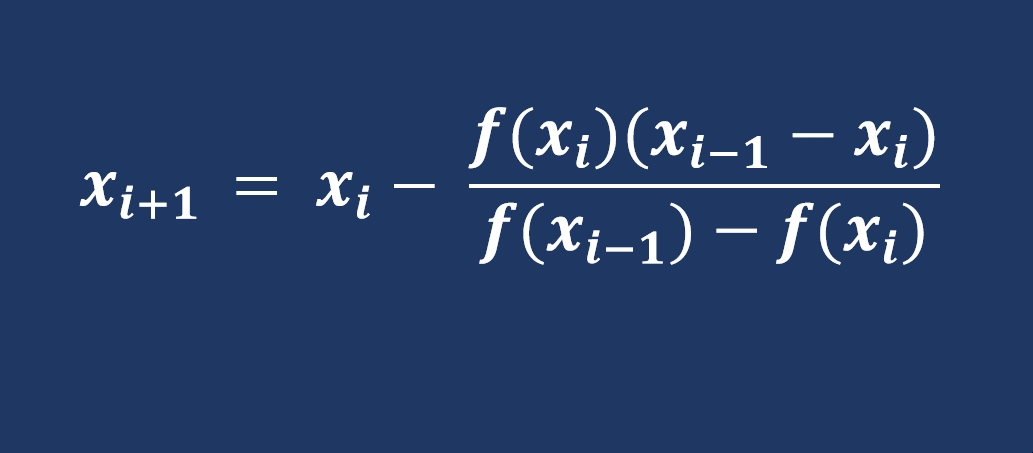
In contrast to the Regula-Falsi method, the Secant method does not bracket the root and it is not even necessary to bracket the root to start the iteration.
Hence, it is obvious that the iteration may not always coverage. On the other hand, it generally converges faster.
Algorithm for Secant Method
Step 1: Choose i=1
Step 2: Start with the initial guesses, xi-1 and xi
Step 3: Use the formula
Step 4: Find Absolute Error,|Ea|= |(Xi+1 -Xi)/Xi+1|*100
Check if |Ea| <= Es (Prescribed tolerance)
If true then stop
Else go to step 2 with estimate Xi+1, Xi
Secant Method C++ Program
Secant Method C++ Program for function f(x) = x2-2x-5
//Program: Secant Method using C++
//techindetail.com
#include<iostream>
#include<cmath>
//for specifying format
#include<bits/stdc++.h>
using namespace std;
//prespecified error tolerance
#define Es 0.0001
float F(float x){
float res;
res=(x*x)-(2*x)-5;
return res;
}
int main(){
float x0,x1,x2,x2old,f0,f1,f2;
int itr=1;
float Ea;
cout<<"Enter the intial guesses (x0,x1): ";
cin>>x0>>x1;
cout<<"Itr No.\tx0\tx1\tf0\tf1\tx2\t f2\tEa\n";
cout<<"----------------------------------------------------------------------------\n";
while (1){
f0=F(x0);
f1=F(x1);
x2=x1-(f1*(x1-x0)/(f1-f0));
f2=F(x2);
//finding absolute error
Ea=fabs((x2-x1)/x2);
cout<<fixed<<setprecision(3);
cout<<itr<<"\t"<<x0<<"\t"<<x1<<"\t"<<f0<<"\t"<<f1<<"\t"<<x2<<"\t"<<f2<<"\t"<<Ea<<"\n";
x0=x1;
x1=x2;
itr++;
if(Ea<=Es)
break;
}
cout<<"Approximate root (x2) is: "<<x2;
return 0;
}
Code language: C++ (cpp)
Output:
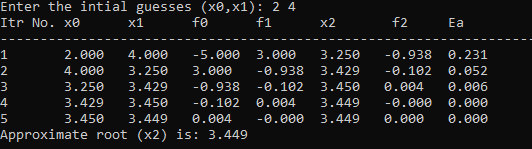
Secant Method Example
Related Numerical Methods: