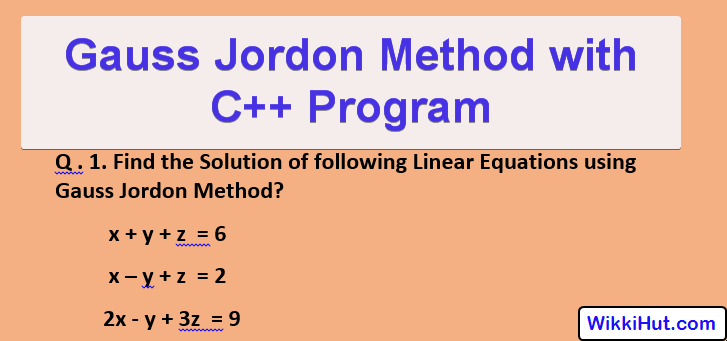
Gauss Jordan Method C++ Program & Example
Gauss Jordan Method C++ is a direct method to solve the system of linear equations and for finding the inverse of a Non-Singular Matrix.
This is a modification of the Gauss Elimination Method.
In this method, the equations are reduced in such a way that each equation contains only one unknown exactly at the diagonal place.
Thus the system of equations are reduced in the special diagonal matrix called identity matrix. It gives us the exact value of variables.
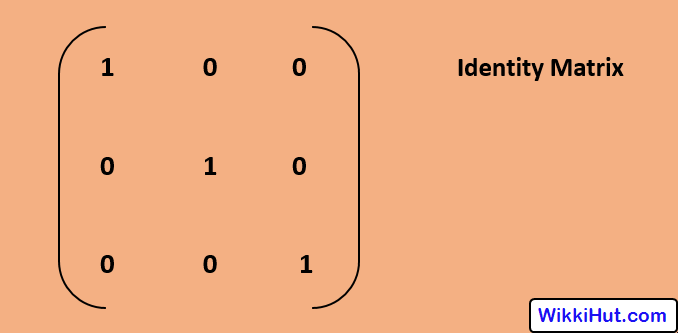
Example
Ex. Find the Solution of following Linear Equations using the Gauss Jordan Method?
x + y + z = 6
x – y + z = 2
2x – y + 3z = 9
Sol: The solution is obtained by reducing the Augmented [Ab] matrix into the diagonal or identity matrix.
Note: We can Perform the Elementary Row Operations only.
Step 1: Write Equations in the form of AX=b, i.e. Matrix Form.
Where,
A = Coefficient Matrix,
X = variables (Column Matrix),
B = constants (Column Matrix.
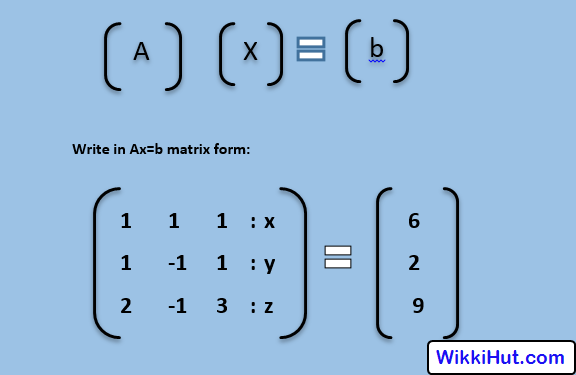
Step 2: Find Augmented Matrix C = [ Ab ]
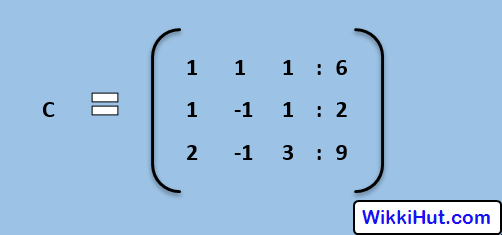
Step 3: Transform Augmented Matrix [C=Ab] into Identity Matrix.
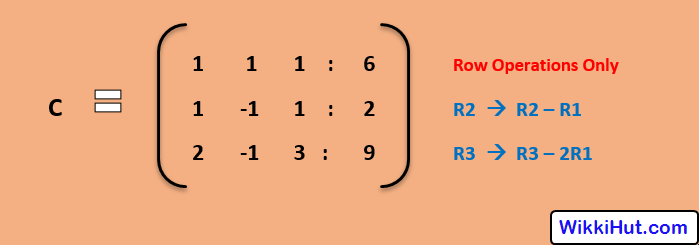
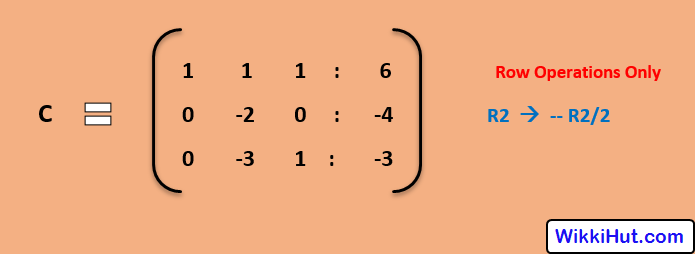
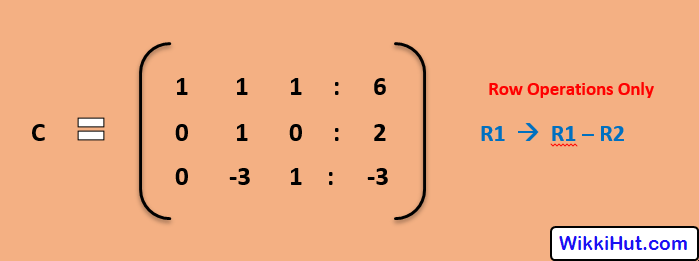
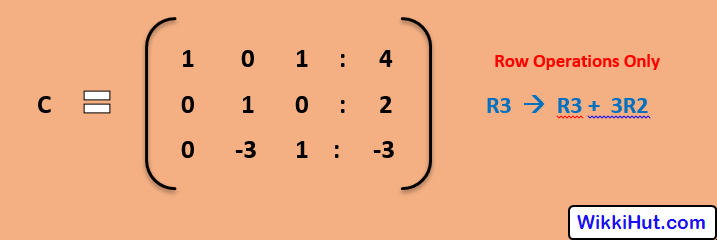
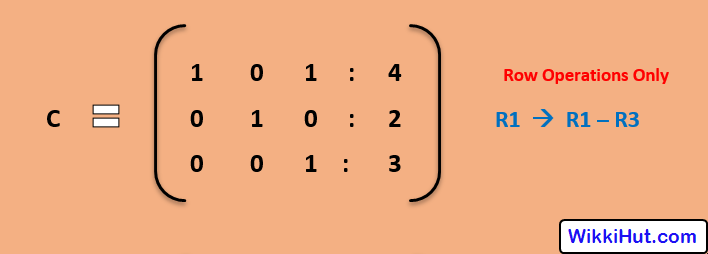
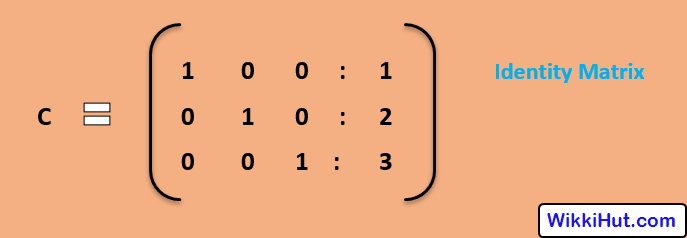
Gauss Jordan Method C++ Program
//Gauss Jordan Method C++ code
//techindetail.com
#include<iostream>
#include<iomanip>
#include<cmath>
#define N 3
using namespace std;
int main()
{
float Matrix[N][N+1],x[N];
// Matrix = Augumented Matrix [Ad]
float temp;
//variables for loops
int i,j,k;
//Scan values of Matrix.
cout<<"Enter Elements of "<<N<<" Rows & "<<N+1<<" Columns\n";
for(i=0; i<N; i++)
{
cout<<"\tEnter Row "<<i+1<<" & Press Enter\n";
for(j=0; j<N+1; j++)
cin>>Matrix[i][j];
}
//make above matrix Identity or Diagonal Matrix
cout<<fixed;
for(j=0; j<N; j++)
for(i=0; i<N; i++)
if(i!=j)
{
temp=Matrix[i][j]/Matrix[j][j];
for(k=0; k<N+1; k++)
Matrix[i][k]-=Matrix[j][k]*temp;
}
//print the Diagonal matrix
cout<<"\n ---------------------------------\n";
cout<<"\n Diagonal Matrix is:\n";
for(i=0; i<N; i++)
{
for(j=0; j<N+1; j++)
cout<<setw(8)<<setprecision(4)<<Matrix[i][j];
cout<<endl;
}
cout<<"\n ---------------------------------\n";
//print values of x,y,z
cout<<"\n The Solution is:\n";
for(i=0; i<N; i++)
cout<<"x["<<setw(3)<<i+1<<"]="<<setw(7)<<setprecision(4)<<Matrix[i][N]/Matrix[i][i]<<endl;
//techindetail.com/gauss-jordan-c/
return 0;
}
Code language: C++ (cpp)
Note:-
For a system of 10 equations, the number of multiplications required for the Gauss Jordan method is about 500.
Whereas for the Gauss Elimination method we need only 333 multiplications.
So the Gauss Elimination method is preferred over the Gauss Jordan method especially in large no of equations.
Suggested Read:
See Wikipedia for More