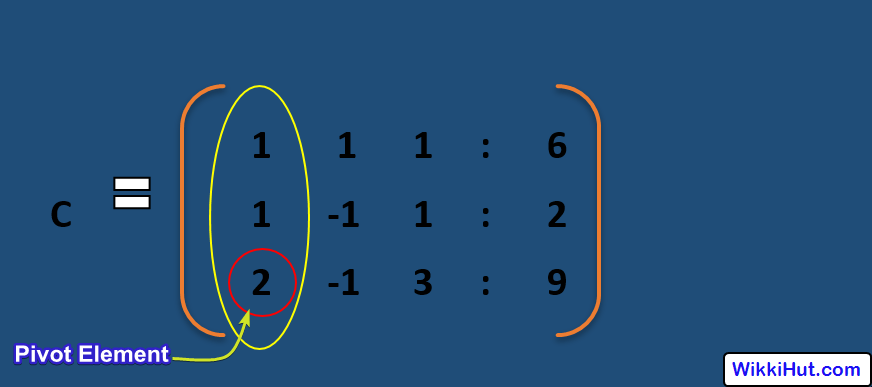
Intro: Gauss Elimination with Partial Pivoting
Gauss Elimination with Partial Pivoting is a direct method to solve the system of linear equations.
In this method, we use Partial Pivoting i.e. you have to find the pivot element which is the highest value in the first column & interchange this pivot row with the first row.
Then you can use the normal Gauss Elimination method to transform the Augmented Matrix into the upper triangular matrix.
Why Partial Pivoting?
Gauss elimination has a limitation where it fails to show exact solution.
- Division by Zero
- Round Off Errors
So to overcome this problem we use Partial Pivoting with Gauss elimination.
Ex. Find the Solution of following Linear Equations using Gauss Elimination with Partial Pivoting?
x + y + z = 6
x – y + z = 2
2x – y + 3z = 9
Step 1:- Write the given System of Equations in the form of AX=b, i.e. Matrix Form.
Where,
A = Coefficient Matrix,
X = variables (Column Matrix),
B = constants (Column Matrix.
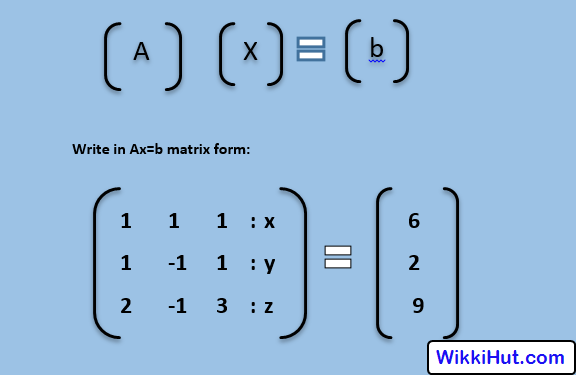
Step 2:- Find Augmented Matrix C = [ Ab ]
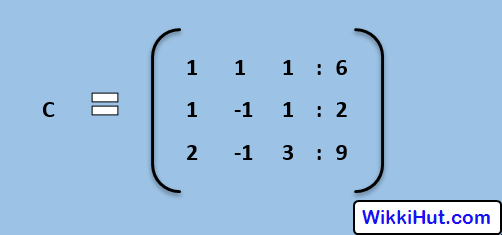
Step 3: Find the Pivot Element.
- Select Largest Absolute Value from 1st Column.
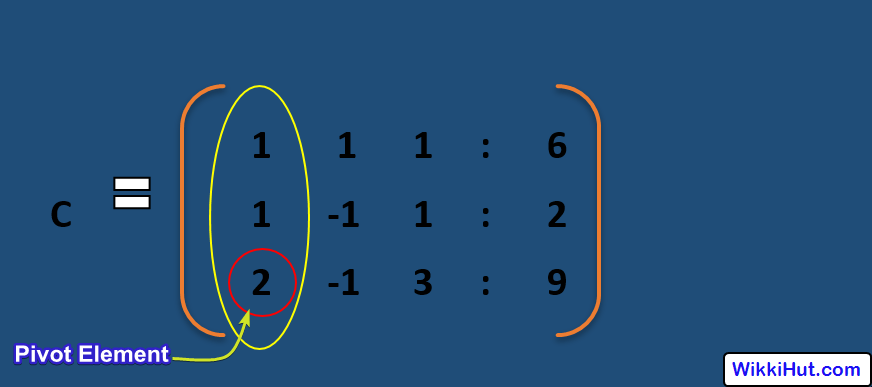
2. Interchange Pivot Row with 1st Row.
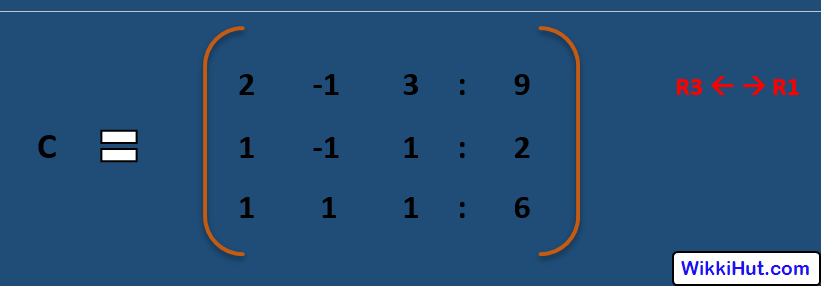
3. Now 1st element ( Pivot element ) should be 1.
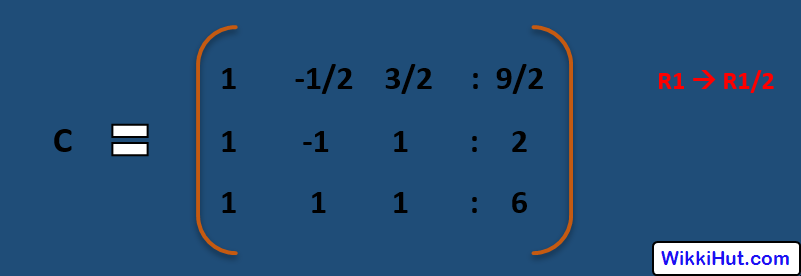
Step 4: Transform into Upper Triangular Matrix Form ( Echelon ).
Echelon: Upper Triangular Matrix with Diagonal Elements 1 or Non-zero.
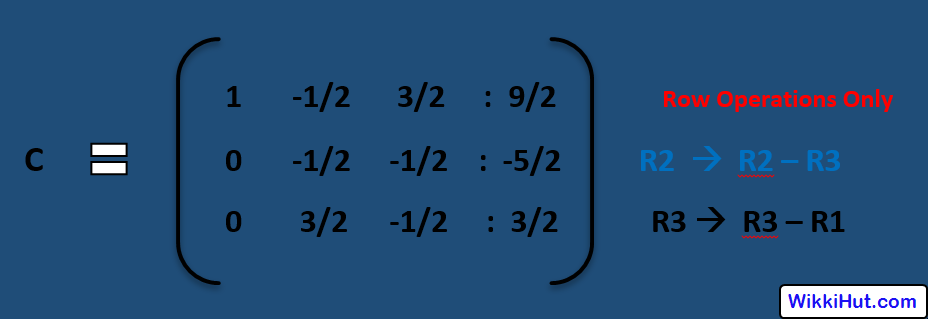
- Repeat Step 3 in Sub Matrix. Find New Pivot Element.
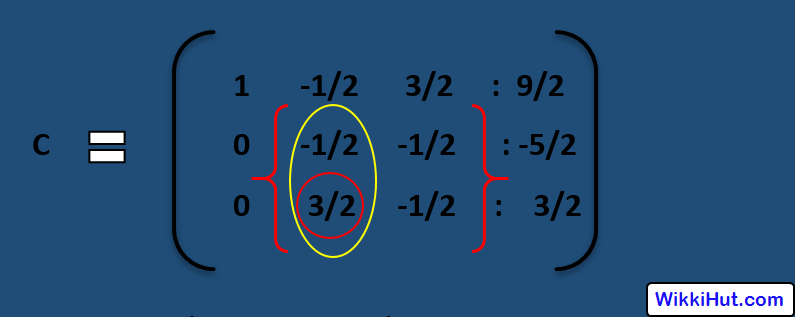
2. Interchange R2 with R3 ( pivot row).
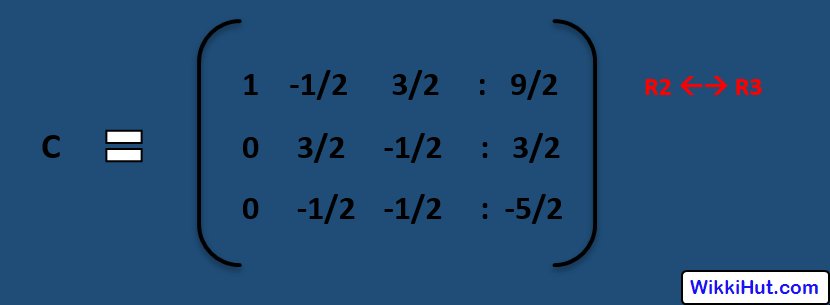
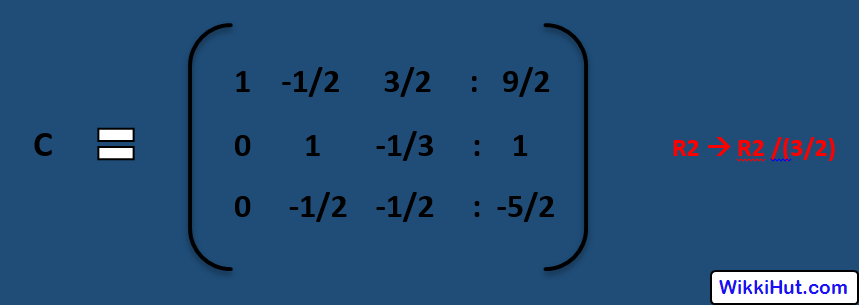
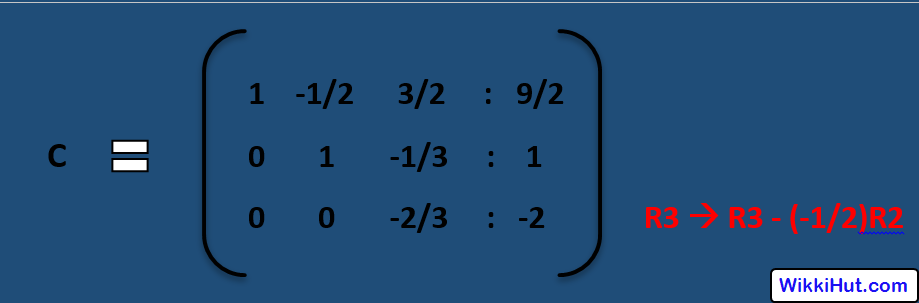
Step 5: Using Back Substitution Find x,y,z.
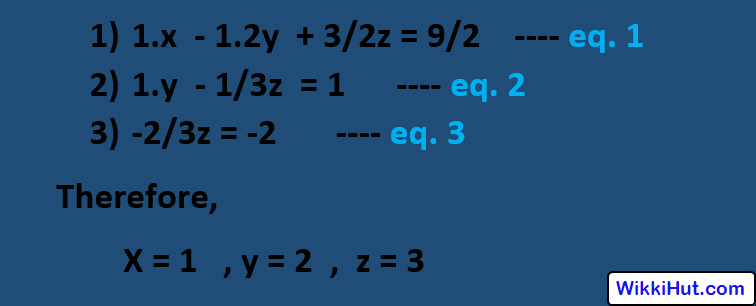
C++ Program Partial Pivoting
//gauss elimination Partial Pivoting
//techindetail.com
#include<iostream>
#include<stdio.h>
#include<conio.h>
#include<math.h>
using namespace std;
int main()
{
int n,i,j,k,temp;
float a[10][10],c,d[10]={0};
cout<<"Enter No of equations ? ";
cin>>n;
cout<<"Coefficient of all Variables : \n";
for(i=0;i<n;i++)
{
cout<<"equation: "<<i+1<< " ";
for(j=0;j<=n;j++)
cin>>a[i][j];
}
// partial pivoting
for(i=n-1;i>0;i--)
{
if(a[i-1][0]<a[i][0])
for(j=0;j<=n;j++)
{
c=a[i][j];
a[i][j]=a[i-1][j];
a[i-1][j]=c;
}
}
//DISPLAY MATRIX
for(i=0;i<n;i++)
{
for(j=0;j<=n;j++)
printf("%6.1f",a[i][j]);
printf("\n");
}
//changing to upper triangular matrix
//Forward elimination process
for(k=0;k<n-1;k++)
for(i=k;i<n-1;i++)
{
c= (a[i+1][k]/a[k][k]) ;
for(j=0;j<=n;j++)
a[i+1][j]-=c*a[k][j];
}
// DISPLAYING UPPER TRIANGULAr MATRIX
printf("\n\n");
for(i=0;i<n;i++)
{
for(j=0;j<=n;j++)
printf("%6.1f",a[i][j]);
printf("\n");
}
//Backward Substitution method
for(i=n-1;i>=0;i--)
{
c=0;
for(j=i;j<=n-1;j++)
c=c+a[i][j]*d[j];
d[i]=(a[i][n]-c)/a[i][i];
}
// DISPLAY
for(i=0;i<n;i++)
cout<<d[i]<<endl;
getch();
return 0;
//techindetail.com
}
Code language: C++ (cpp)
Other Related Numerical Methods.
- Gauss Elimination Method
- Gauss-Seidel Method
- Bisection Method C++
- Newton Raphson Method
- Gauss Jordon Method
- Regula Falsi Method C++