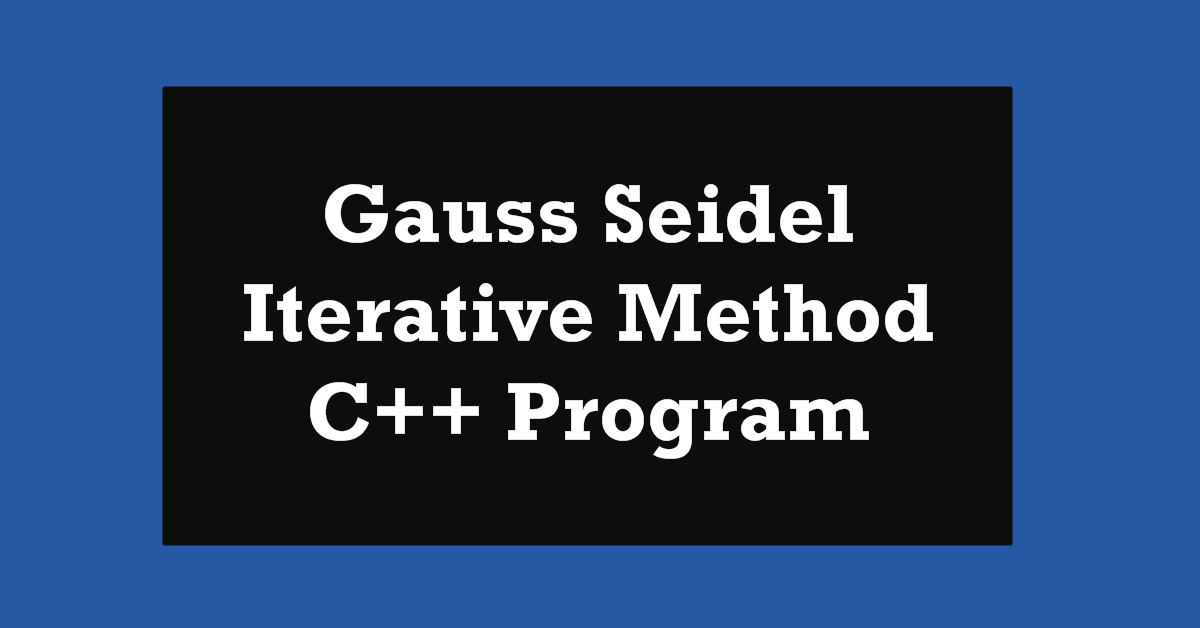
Gauss seidel-iterative method c++
Iterative or approximate methods provide an alternative to the elimination methods described to this point.
Those approaches consisted of guessing a value and then using a systematic method to obtain a refined estimate of the root.
The Gauss-Seidel method is the most commonly used iterative method. read more
Must Read: Lu Decomposition Method C++
Gauss Seidel-iterative method C++ Program
//Gauss-seidel Iterative method
//techindetail.com
#include<iostream>
#include<conio.h>
using namespace std;
int main(void)
{
float a[10][10], b[10], x[10], y[10];
int n = 0, m = 0, i = 0, j = 0;
cout << "Enter size of 2d array(Square matrix) : ";
cin >> n;
for (i = 0; i < n; i++)
{
for (j = 0; j < n; j++)
{
cout << "Enter values no :(" << i << ", " << j << ") ";
cin >> a[i][j];
}
}
cout << "\n Enter Values to the right side of equation\n";
for (i = 0; i < n; i++)
{
cout << "Enter values no :(" << i << ", " << j << ") ";
cin >> b[i];
}
cout << "Enter initial values of x\n";
for (i = 0; i < n; i++)
{
cout << "Enter values no. :(" << i<<"):";
cin >> x[i];
}
cout << "\nEnter the no. of iteration : ";
cin >> m;
while (m > 0)
{
for (i = 0; i < n; i++)
{
y[i] = (b[i] / a[i][i]);
for (j = 0; j < n; j++)
{
if (j == i)
continue;
y[i] = y[i] - ((a[i][j] / a[i][i]) * x[j]);
x[i] = y[i];
}
printf("x%d = %f ", i + 1, y[i]);
}
cout << "\n";
m--;
}
return 0;
}
Code language: C++ (cpp)
C++ program Gauss-Seidel method.
Read More: Gauss Jordan Method C++ Example
Example | Gauss Siedel Method
We will first illustrate the Gauss-Seidel method on two simultaneous equations.
Later we will generalize it to n equations in n unknowns.
Consider the simultaneous equations given below:
x1 + x2= 2 (1)
3×1-10×2= 3 (2)
Start with an initial value of x2=0. Substitute this in Equation (1) and obtain the value of x1=2.
Use this guessed value in Equation (2) to obtain a refined guess for x2. This is given by x2 = (3×1-3)/10 = (6-3)/10= .3
Use this in Equation (1) to get another value of x1. The progress of the iterations is shown in Table (1)
Iteration no. | X1 | X2 |
---|---|---|
1 | 2 | 3 |
2 | 1.7 | .21 |
3 | 1.79 | .237 |
4 | 1.763 | .229 |
5 | 1.771 | .231 |
6 | 1.769 | .231 |
7 | 1.769 | .231 |
Observe that the values of x1 and x2 converge to 1.769 and .231 respectively which is the solution.
As this is an iterative method iterations are stopped when successively values of x1 as well as of x2 are “closer enough”.
This is a successive approximations method and the technique is illustrated graphically in figure (1).
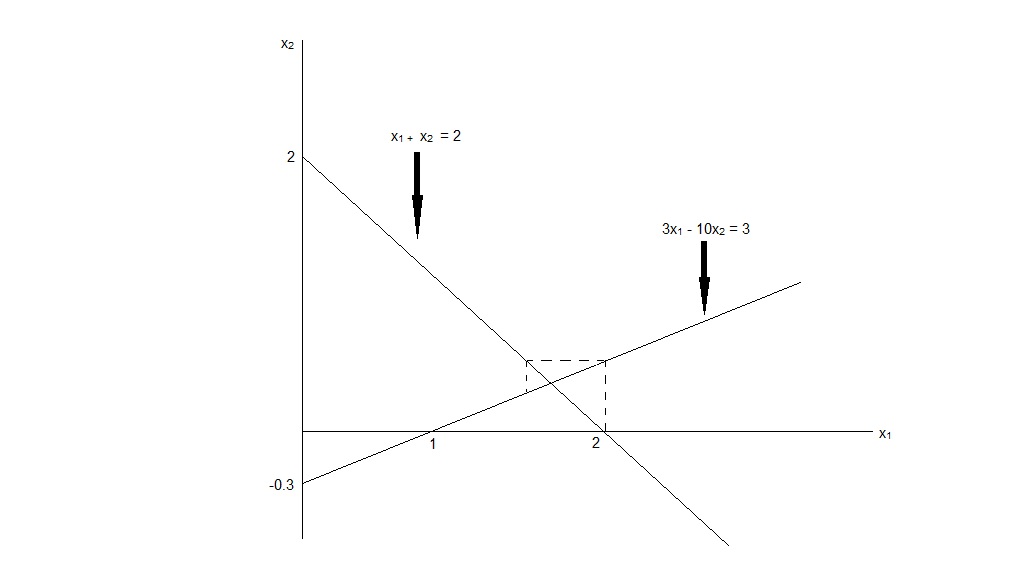
Related: Regula Falsi Method Solved Example
Generalized form:
Now let’s generalize Gauss-seidel Iterative method
Assume that we are given a set of n equations:
[A]{X}={B}
Suppose that for conciseness we limit ourselves to a 3 × 3 set of equations.
If the diagonal elements are all nonzero, the first equation can be solved for x1, the second for x2, and the third for x3 to yield
x1 =(b1 − a12x2 − a13x3)⁄a11 2(a)
x2 =(b2 − a21x1 − a23x3)⁄a22 2(b)
x3 =(b3 − a31x1 − a32x2)⁄a33 3(b)
Now, we can start the solution process by choosing guesses for the x’s. A simple way to obtain initial guesses is to assume that they are all zero. These zeros can be substituted into Eq. (2a), which can be used to calculate a new value for
x1 = b1/a11
Then, we substitute this new value of x1 along with the previous guess of zero for x3 into Eq. (2b) to compute a new value for x2.
The process is repeated for Eq. (2c) to calculate a new estimate for x3. Then we return to the first equation and repeat the entire procedure until our solution converges closely enough to the true values. Convergence can be checked using the criterion
|εa,i| = |xij − xij-1|/|xij|× 100% < εs 2(c)
for all i, where j and j − 1 are the present and previous iterations.
Related: Bisection Method Solved Example
Algorithm:
- for i=1 to n in steps of 1 and j=1 to n+1 in steps of 1 do read aij end for.
- Read e, maxit
Note: e is the allowed relative error in the result vector. maxit is maximum number of iterations allowed for the solution to converge. - for i=1 to n in steps of 1 xi=0 end for
- for itr=1 to maxit do
- big=0
- for i=1 to n in steps of 1 do
- sum=0
- for j=1 to n in steps of 1 do
- for (j≠i) then sum =sum+aijxj end for
- temp=(ai(n+1)-sum)/aii
- relerror=|(xi-temp)/temp|
- if relerror > big then big=relerror
- xi=temp
end for - if (big<=e) then
begin Write ‘converges to a solution’
for i=1 to n in steps of 1 do Write xi endfor
stop end
end for - Write ‘Does nott converge in maxit iteratioins’
- for i=1 to n in steps of 1 do Write xi end for
- Stop
This is the Algorithm for Gauss-Seidel Iterative method C++