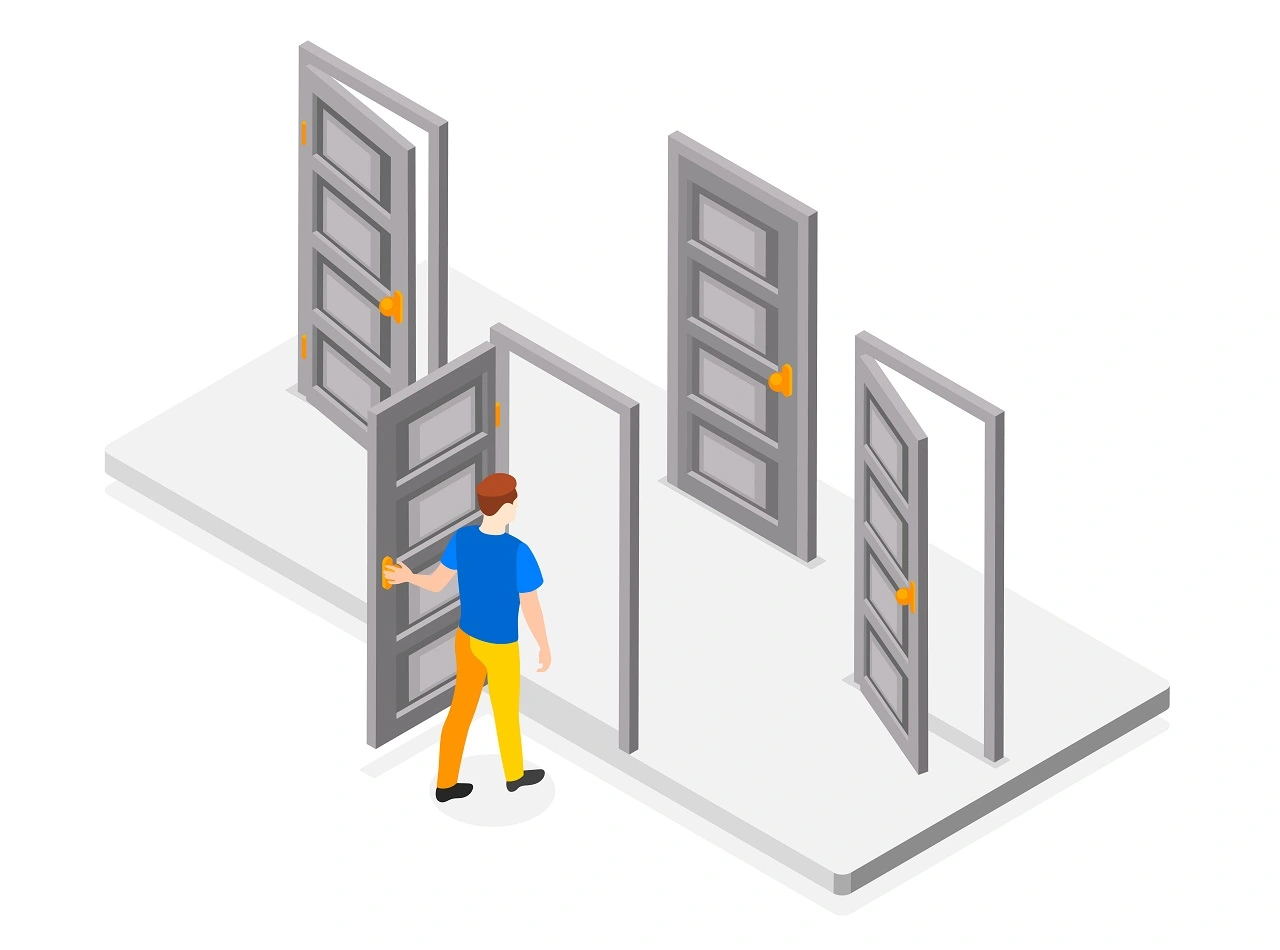
The 6 different ways to redirect users using JavaScript from one web page to another are window.location.href, window.location.replace(), window.location.assign(), changing window.location directly, and using HTML Anchor tags.
Here’s a list of all the JavaScript Redirect Methods discussed in the article:
window.location.href
window.location.replace()
window.location.assign()
- Changing
window.location
- Using an HTML anchor tag
- Opening a new window or tab using
window.open()
These methods provide different ways to redirect users from one web page to another or from one URL to another URL using JavaScript.
Here are two versions of code snippets for each method – one without a delay (simple version) and another with a delay (using setTimeout()
), along with different use cases for each method:
JavaScript Redirect Methods
Method 1: window.location.href
The window.location.href
property is a widely used method for redirecting users.
It allows you to set the URL of the current window to a new location. Here’s an example:
Simple Version:
// Simple version without delay
window.location.href = "https://www.example.com";
Code language: JavaScript (javascript)
Delayed Version with Use Case:
// Delayed version with a use case
setTimeout(function() {
window.location.href = "https://www.example.com";
}, 5000);
Code language: JavaScript (javascript)
Use Case 1:
You can use this method to redirect users to a landing page or promotional offer after a specific time delay.
For example, if you want to redirect users to a limited-time discount offer after 5 seconds of visiting your website.
Use Case 2:
You can use this method to show a “Thank you” message to the user for completing a form submission, and then automatically redirect them to a confirmation page after a certain delay.
Method 2: window.location.replace()
The window.location.replace()
method is similar to window.location.href
, but with a slight difference in behavior.
Instead of adding the new page to the browser history, it replaces the current page, preventing the user from navigating back.
Simple Version:
// Simple version without delay
window.location.replace("https://www.example.com");
Code language: JavaScript (javascript)
Delayed Version with Use Case:
// Delayed version with a use case
setTimeout(function() {
window.location.replace("https://www.example.com");
}, 5000);
Code language: JavaScript (javascript)
Use Case 1: Let’s say you want to redirect users to a login page after they successfully sign out. By replacing the current page with the login page URL, you ensure that users cannot go back to the previous authenticated page.
Real-life use case: You can use this method to implement a timed logout functionality.
For example, if the user is inactive for a certain period of time, you can display a warning message and automatically log them out by replacing the current page with the logout URL after a specific delay.
|Related: TOC using JavaScript
Method 3: window.location.assign()
Another way to redirect users is by using the window.location.assign()
method.
It adds the new URL to the browser history, allowing users to navigate back using the back button.
Simple Version:
// Simple version without delay
window.location.assign("https://www.example.com");
Code language: JavaScript (javascript)
Delayed Version with Use Case:
// Delayed version with a use case
setTimeout(function() {
window.location.assign("https://www.example.com");
}, 5000);
Code language: JavaScript (javascript)
Use Case 1: Suppose you have a multi-step registration process, and after users complete a step, you want to redirect them to the next step after a few seconds.
By using the delayed version, you can ensure a smooth transition between registration steps.
Real-life use case: Suppose you have an e-commerce website with a “Continue Shopping” button.
After the user adds an item to their cart, you can show a notification message and automatically redirect them to the product listing page with a delay.
Method 4: Changing window.location
directly
A simpler and more concise way to redirect users is by assigning the URL directly to window.location
.
Simple Version:
// Simple version without delay
window.location = "https://www.example.com";
Code language: JavaScript (javascript)
Delayed Version with Use Case:
// Delayed version with a use case
setTimeout(function() {
window.location = "https://www.example.com";
}, 5000);
Code language: JavaScript (javascript)
Use Case: Imagine you have a website that provides different language options.
You can use this method to redirect users to the appropriate language version based on their browser settings after a certain delay.
Real-life use case: Let’s say you have a promotional landing page that displays a countdown timer.
You can set up the page to automatically redirect to a different page, such as the product page or a special offer page, once the countdown reaches zero.
Method 5: Using an HTML anchor tag
If you have an HTML anchor tag (<a>
) and want to simulate a click to redirect users, you can achieve it using JavaScript.
Simple Version:
<a href="https://www.example.com">Redirect</a>
Code language: HTML, XML (xml)
Delayed Version with Use Case:
<a href="https://www.example.com" id="redirectLink">Redirect</a>
<script>
setTimeout(function() {
document.getElementById("redirectLink").click();
}, 5000);
</script>
Code language: HTML, XML (xml)
Use Case 1: Consider a scenario where you have a “Read More” link that expands content on the page.
By using the delayed version, you can automatically simulate a click after a few seconds to provide a seamless reading experience.
Real-life use case 2: Consider a scenario where you have a social media sharing button on your website.
By automatically triggering a click event after a delay, you can encourage users to share content on their social media platforms.
Method 6: Opening a new window or tab
In some cases, you may want to open a new browser window or tab with the desired URL.
JavaScript provides the window.open()
method for this purpose.
Simple Version:
// Simple version without delay
window.open("https://www.example.com", "_blank");
Code language: JavaScript (javascript)
Delayed Version with Use Case:
// Delayed version with a use case
setTimeout(function() {
window.open("https://www.example.com", "_blank");
}, 5000);
Code language: JavaScript (javascript)
Real-life use case: Suppose you have a web application that generates reports.
You can provide users with an option to open the report in a new window or tab with a specific size and position for better visibility and ease of use.
|Also Read: Nested List using JS
Conclusion
Redirecting users using JavaScript is a powerful feature that allows you to enhance the user experience and guide them to relevant content.
Whether you need a simple instant redirect or a delayed redirect with additional parameters, JavaScript provides a range of methods to suit your needs.
By understanding the different approaches and their use cases, you can leverage these methods effectively in your web applications.
Experiment with these techniques and choose the one that best fits your specific requirements, making the redirection process seamless and intuitive for your users.
FAQ’s
Q: Can I pass parameters or query strings when redirecting using JavaScript?
A: Yes, you can include parameters or query strings in the URL when redirecting.
For example, you can add ?param1=value1¶m2=value2
at the end of the URL to pass data to the new page.
Q: How can I delay the redirection using JavaScript?
A: You can use the setTimeout()
function to introduce a delay before redirecting the user. For example, setTimeout(function() { window.location.href = "https://www.example.com"; }, 5000)
will redirect the user after a 5-second delay.
Q: What is the difference between window.location.replace()
and window.location.href
?
A: The main difference is that window.location.replace()
replaces the current page in the browser history, preventing the user from navigating back, while window.location.href
adds the new page to the history, allowing the user to navigate back.
Q: Can I open the redirected URL in a new window or tab?
A: Yes, you can open the redirected URL in a new window or tab using the window.open()
method.
For example, window.open("https://www.example.com", "_blank")
will open the URL in a new tab.
Q: Can I simulate a click on an HTML element to trigger a redirection?
A: Yes, you can programmatically simulate a click on an HTML element, such as an anchor tag, to redirect users using JavaScript.
For example, document.getElementById("redirectLink").click()
will trigger the click event on the element with the ID “redirectLink” and redirect the user.
Q: Are there any restrictions or considerations when redirecting users with JavaScript?
A: When redirecting users, ensure that you have permission to redirect them to the target URL. Also, consider the impact on the user experience, especially when introducing delays,