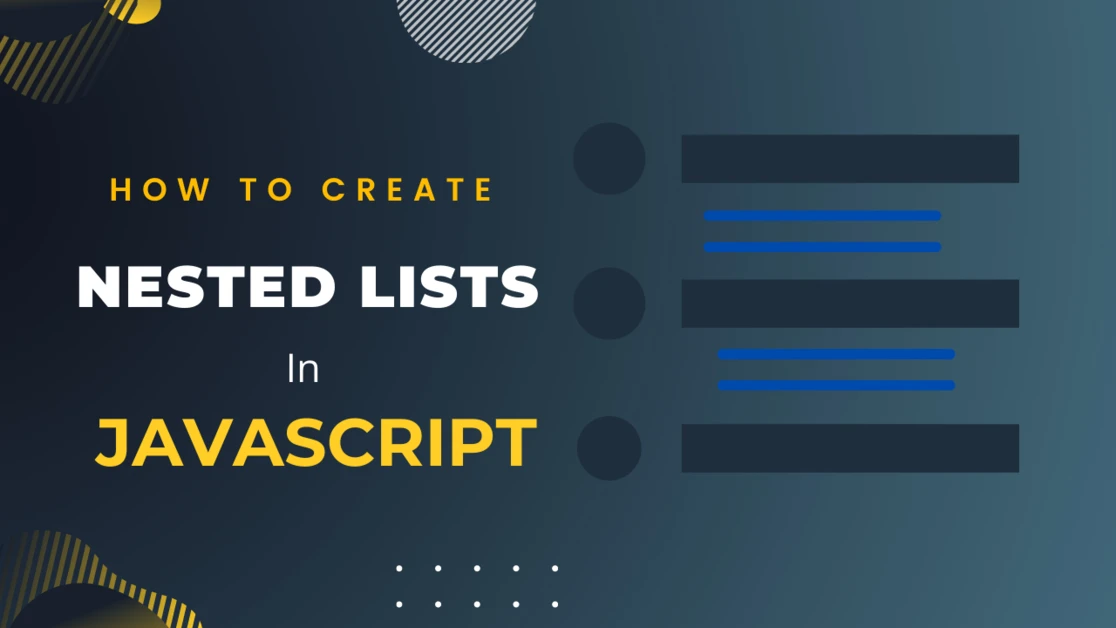
An Html list inside another Html list is called a nested list. The nested list can be OL inside the UL or OL and vice versa.
In Html making a nested list is very easy, but in JavaScript, it is a lit bit tricky.
In this Tutorial, you will learn how to create a nested list in JavaScript dynamically.
1. HTML Structure
For this tutorial, you only need a simple blank Html page in which you will add a nested list using javascript.
Html Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title> Nested List JavaScript </title>
</head>
<body>
// Content goes here
</body>
<html>
Code language: HTML, XML (xml)
2. CSS Style
To make our page a lit bit attractive add some basic CSS properties. Our focus is only on javascript.
<style>
body {
min-height: 100vh;
display: flex;
justify-content: center;
align-items: center;
background-color: aliceblue;
font-family: 'Open Sans', Arial;
font-size: 18px;
}
ul {
outline: 2px solid #ddd;
padding: 1rem 2rem;
border-radius: 0.5rem;
list-style-position: inside;
color: blue;
}
::marker {
color: #747474;
}
ul ol {
font-size: .9em;
margin: 0.4rem 0;
}
</style>
Code language: HTML, XML (xml)
3. JavaScript Code
Take a look at the following javascript code that will dynamically make Html nested lists and insert them into our Html page.
Following are the steps to create a nested list in JavaScript.
- Create a Div
- Make few variables
- Create Objects of Data
- Use Loop
- Create Lists
<script>
let div = document.createElement('div');
document.body.appendChild(div);
let ul = document.createElement('ul');
let li = null;
let subLi = null;
let skills = {
FrontEnd: ['Html', 'Css', 'JavaScript', 'React'],
BackEnd: ['NodeJs', 'ExpressJs'],
Database: ['MongoDB', 'MySql']
};
const values = Object.entries(skills);
values.forEach(([key, value]) => {
let li = document.createElement('li');
li.innerText = key;
let subOl = document.createElement('ol');
for (let i in value){
let subValue = value[i];
subLi = document.createElement('li');
subLi.innerText = subValue;
subOl.appendChild(subLi);
}
li.appendChild(subOl);
ul.appendChild(li);
});
div.appendChild(ul);
</script>
Code language: HTML, XML (xml)
Create a Div
Let us create a div that will be the container for the lists.
let div = document.createElement('div');
Code language: JavaScript (javascript)
Make few variables
Create a UL element that will be the main list. Also, make variables for the li items.
let ul = document.createElement('ul');
let li = null;
let subLi = null;
Code language: JavaScript (javascript)
Create Object of Data
Here the variable skills
contains the actual data which you will convert into Html nested lists.
It is the object of values, in the form of key and value pairs. The values on the left-hand side will become the main list items and values on the right side will become the nested list of its parent element.
let skills = {
FrontEnd: ['Html', 'Css', 'JavaScript', 'React'],
BackEnd: ['NodeJs', 'ExpressJs'],
Database: ['MongoDB', 'MySql']
};
Code language: JavaScript (javascript)
Now you need to convert the skills
object into the array data structure to perform a simple iteration.
The const values
now contains an array of arrays.
const values = Object.entries(skills);
Code language: JavaScript (javascript)
Use forEach
Loop
Now start the forEach loop, this loop will iterate over all the items of the array values
.
values.forEach(([key, value]) => {
// body of loop
}
);
Code language: JavaScript (javascript)
Inside the loop create li items of the main list. The value inside the li will be the key that is data on the left-hand side of the skills
object.
let li = document.createElement('li');
li.innerText = key;
Code language: JavaScript (javascript)
Also, make a sub-list, it will be used next.
let subOl = document.createElement('ol');
Code language: JavaScript (javascript)
Use for Loop
Now to make a sub-list you need to use another loop to iterate all the values of the array.
Inside this loop create a li item for every value of the array and append these li items to the sub list declared earlier.
for (let i in value){
let subValue = value[i];
subLi = document.createElement('li');
subLi.innerText = subValue;
subOl.appendChild(subLi);
}
Code language: JavaScript (javascript)
Outside the inner loop append the sub list to the main li item and finally append the main li to the main list UL.
li.appendChild(subOl);
ul.appendChild(li);
Code language: CSS (css)
The final step now is to append the Ul to the Div container outside the main loop.
div.appendChild(ul);
Code language: CSS (css)
Related: Format Javascript Strings
Output Nested List
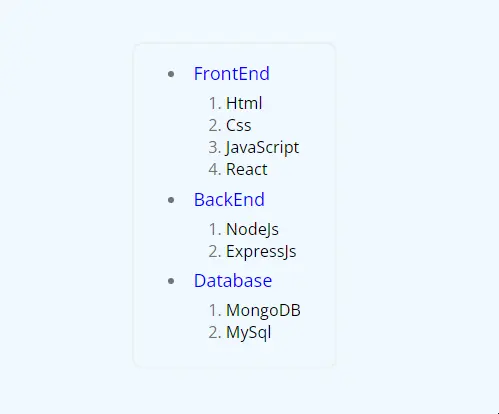
End of Tutorial, Thank You!
Also Read: Create Table of Contents using Javascript