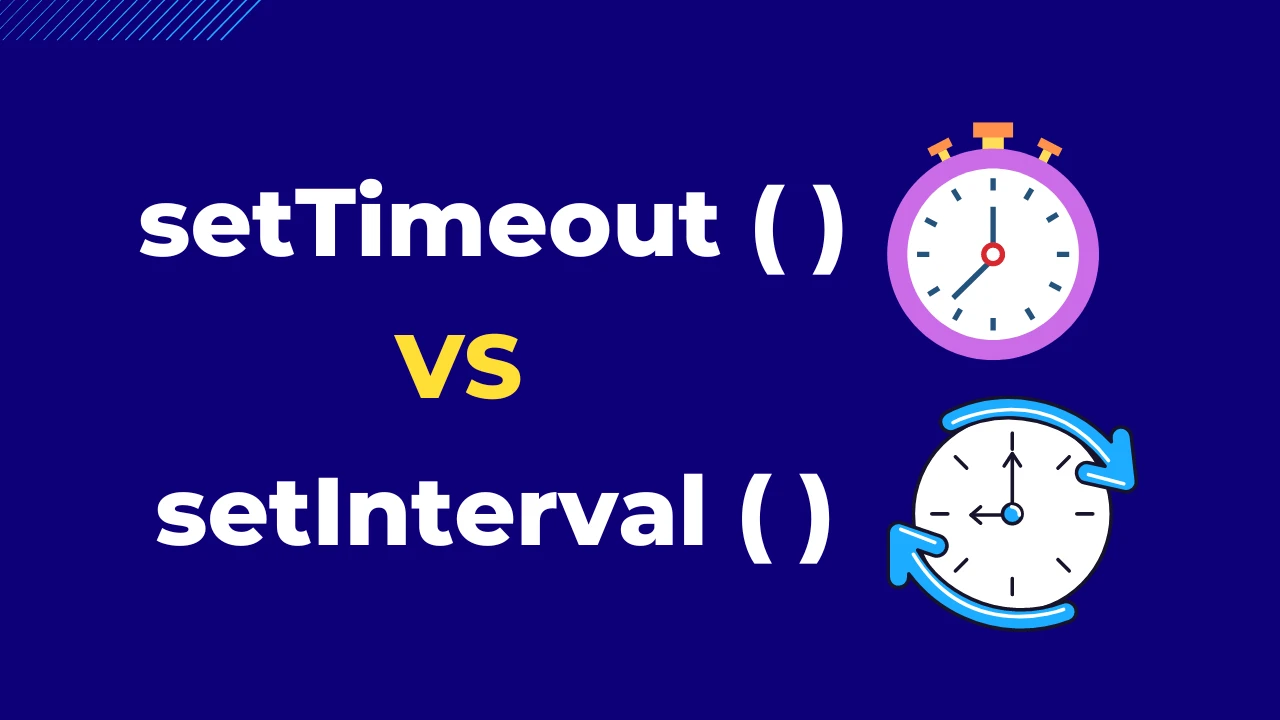
In this article, you will learn the basic concepts of setTimeout () and setInterval () Javascript functions and their differences.
Suppose you need to delay the execution of a certain part of your code, you can use the setTimout()
and setInterval()
functions for that.
The process of delaying the execution of the function is called Scheduling in js.
In JS there are 2 methods to delay:
- setTimeout
- setInterval
Note:- Nested setTimout is more flexible and can be used in place of setInterval.
1. setTimeout
The setTimeout method delays the execution of the code for the particular time passed as the argument to it.
setTimeout() syntax:
let delayFunc = setTimeout(func, [delay], [arg1], [arg2], ...);
Code language: JavaScript (javascript)
setTimout Parameters:
func
The first argument is the function name to be executed, it is not recommended to place the actual code here.
delay
The second argument is the delay time in milliseconds by default it is 0.
Note:- 1 second = 1000 ms.
arg1, arg2, arg3 …
Arguments to be passed to the function, are optional.
setTimeout() Example.
In the following code, suppose we want to display a message after 3 seconds.
For instance, this code calls showMsg()
after 3 seconds.
function showMsg() {
console.log('Welcome');
}
setTimeout(showMsg, 3000);
Code language: JavaScript (javascript)
So, after 3 seconds the above code will output the message in the console.
You can also use setTimout with the arguments as below.
function showMsg(msg, name) {
alert( msg + ', ' + name );
}
setTimeout(showMsg, 2000, "Welcome", "Sam");
// Output: Welcome, Sam
Code language: JavaScript (javascript)
The above code will execute after 2 seconds with the arguments.
Note:- If the first argument is a string, then JavaScript creates a function from it.
So, this will also work:
setTimeout("alert('Welcome')", 2000);
Code language: JavaScript (javascript)
But using strings is not recommended, use arrow functions instead of them, like this:
setTimeout(() => alert('Welcome'), 2000);
Code language: JavaScript (javascript)
clearTimeout()
In some situations, you may need to stop the delayed function set by setTimout by using the clearTimout()
function.
Suppose you have a function named timerFunc
.
let timerFunc = setTimeout(timer, 2000);
Code language: JavaScript (javascript)
The syntax to cancel the setTimout function timerFunc is:
clearTimeout(timerFunc);
Therefore, the above function will not execute.
Zero delay setTimeout
There is a special use case of setTimout method, suppose you may need to execute a block of code after the execution of the current js script.
setTimeout(funcName, 0);
// or just
setTimeout(funcName);
Code language: JavaScript (javascript)
The above two functions schedule the execution of funcName as soon as possible and execute it only after the currently executing script.
Also Read: Format Js Strings
2. setInterval
The setInterval method is also used to delay the code execution but it runs the function regularly after the given interval of time not only once.
setInterval Syntax:
The syntax of the setInterval
method is the same as the setTimeout
method.
let timerFunc = setInterval(func|code, [delay], [arg1], [arg2], …);
Code language: JavaScript (javascript)
All the arguments have the same meaning as the setTimout method.
The only difference is that setTimout runs only once, and setInterval runs repeatedly for the given interval of time.
To stop further calls, we should call clearInterval(timerFunc) same as clearTimout.
setInterval Example.
Suppose you need to show a message after every 2 seconds and stop it after 10 seconds.
The following code will show the message every 2 seconds, And after 6 seconds, the output is stopped.
// repeat after every 2 seconds
let timerFunc = setInterval(showMsg, 2000);
function showMsg(){
alert('ClickTo Subscribe');
}
Code language: JavaScript (javascript)
Or, you can use the arrow function as below.
let timerFunc = setInterval(() => alert('Subscribe'), 2000);
Code language: JavaScript (javascript)
Now, to stop the code after 10 seconds use clearInterval()
method.
// after 6 seconds stop
setTimeout(() => { clearInterval(timeFunc);
alert('stop');
}, 6000);
Code language: JavaScript (javascript)
Nested setTimeout
In JavaScript, there are basically two ways of running something regularly.
- One is setInterval
- Second is Nested setTimout
You can use nested setTimout functions instead of the setInterval function for optimization purposes.
For example:
/** instead of
let timerFunc = setInterval(() => alert('Subscribe'), 2000);
*/
let timerFunc = setTimeout(function subscribe() {
alert('Subscribe');
// nested setTimout
timerFunc = setTimeout(subscribe, 2000);
}, 2000);
Code language: JavaScript (javascript)
The main benefit of the above code is that it schedules the next call right at the end of the current one.
The nested setTimeout is a more flexible method than setInterval.
This way the next call may be scheduled differently, depending on the results of the current one.
Use Cases
Let us say your program (API) needs to fetch the new data from the server after every 10 seconds, and the server is already overloaded.
Then you need to increase the interval time to fetch the data such as 10, 20, …, or 50 seconds.
let delay = 10000;
let timerFunc = setTimeout(function request() {
...send request...
if (if request failed server overload) {
// increase the interval to the next run
delay *= 2;
}
timerFunc = setTimeout(request, delay);
}, delay);
Code language: JavaScript (javascript)
So if the server is overloaded our program will increase the interval time from 10 to 20 seconds, and from 20 to 40 seconds.
Also Read: Create Nested Lists In JS
Conclusion:
The setTimout method delays the code execution for a specific time and the setInterval method also delays the code but it runs the code repeatedly until explicitly stopped.