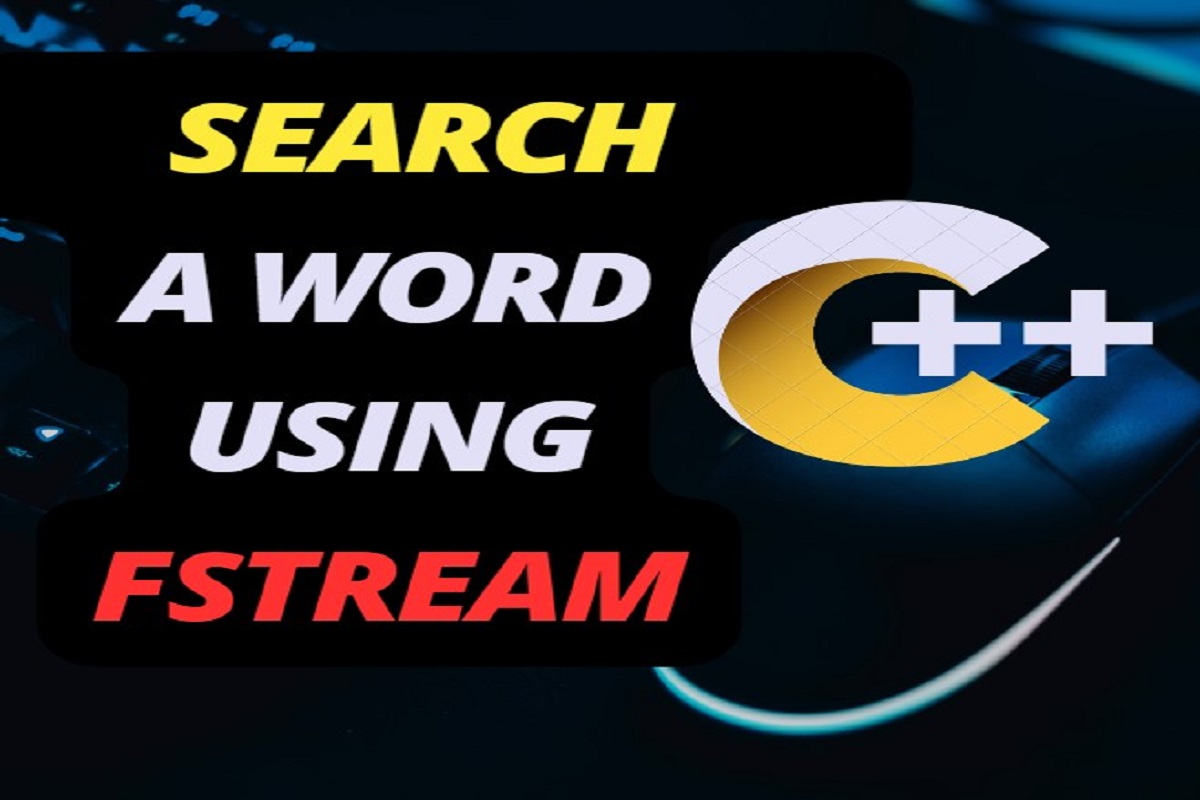
Searching for a Word or a String in a text file in C++ is a very simple task.
It typically involves reading the file first. The process of searching requires comparing the text content or we can say words of the text file with the desired word.
To search for a word in a text file using C++, it is important to first understand how to read text files in C++.
There are several library classes available for read and write operations to files in C++:
- ofstream – To write into a file
- ifstream – To read from the file
- fstream – Both read & write
In this tutorial, you will learn how to search for a word in a text file using a C++ program.
What is fstream
fstream
is a C++ library class that is used for both input and output operations.
It is a part of the <fstream>
header and is used to handle operations like reading from and writing to the files.
The ‘fstream’ class is derived from ‘iostream‘, which in turn is derived from ios class.
This allows the ‘fstream’ class to inherit the functionality and features of both iostream
and ios
classes, providing the methods and operators for opening, closing, reading, and writing files.
C++ Program
Here is a C++ program to search for a word or substring in a text file.
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
// Replace "input.txt" with the name of your text file.
ifstream inputFile("input.txt");
if (!inputFile) {
cerr << "Error opening file." << endl;
return 1;
}
string SearchWord;
cout << "Enter the word to search: ";
cin >> SearchWord;
string word;
int index = 0;
bool found = false;
// reads the file word by word and stores each word.
while (inputFile >> word) {
if (word == SearchWord) {
found = true;
break;
}
index++;
}
if (found) {
cout << "Word found at index: " << index << endl;
} else {
cout << "Word not found." << endl;
}
inputFile.close();
return 0;
}
Code language: PHP (php)
Also Read: How To Compare Strings in C++
Code Explantion
In the above program, we create an ‘fstream’ object named ‘inputfile’ to represent the file in which we want to search for our word.
The ‘inputfile’ object is responsible for performing the reading operation.
We begin by checking whether the file exists and is open or not using the line ‘if (!inputfile)’.
This condition ensures that the file was opened successfully before proceeding with the reading operation.
The line ‘inputfile >> word’ reads the file word by word, extracting one word at a time, and storing it in the word variable.
We can then compare this word with the target word we want to search for in the file.
If the word is found, we can perform further actions, such as printing its index or processing it according to our requirements.
Must Read: Factorial in C++
FAQ’s
How do I check if a file exists before reading it using fstream?
You can check if a file exists by opening it using fstream
and then checking the state of the file stream.
If the file opening is successful, the file exists. You can use the condition if (inputfile) or if (inputfile.is_open())
to verify if the file was opened successfully.
Can I search for a word in a file using ‘fstream’ without reading the entire file?
Yes, it is possible to search for a word in a file using fstream without reading the entire file.
You can read the file line by line or word by word, checking each line or word for the desired word.
Once the word is found, you can perform the necessary actions without continuing to read the rest of the file.
How do I search for a specific word in a text file using ‘fstream‘?
To search for a specific word in a text file using ‘fstream‘, you can read the file word by word using the >>
operator and compare each word with the target word.
If the word matches, you can perform the desired actions such as printing its index or processing it further.