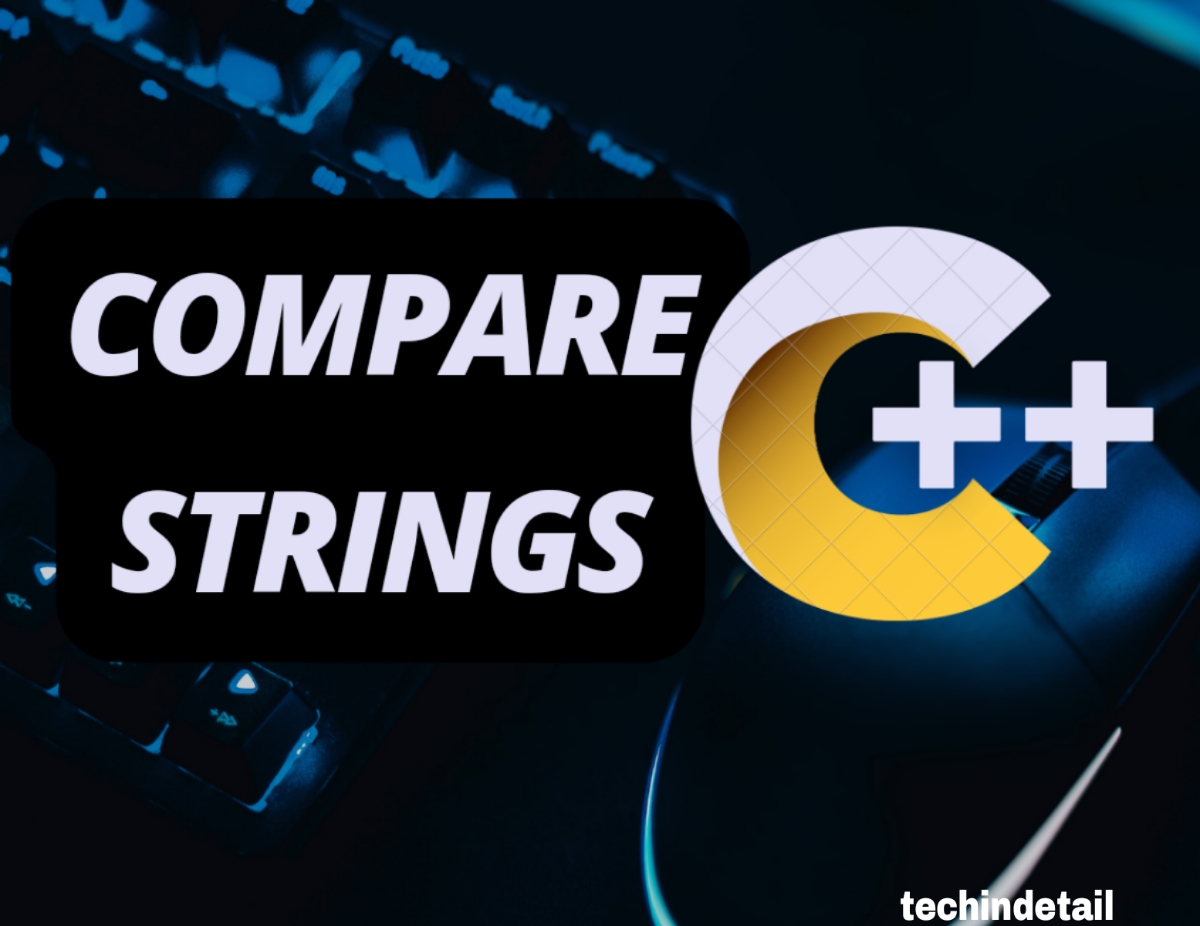
There are basically 3 different ways to compare strings in C++.
strcmp()
function- In-built
compare()
function - C++ Relational Operators
The first one is the strcmp()
function used in the C-string class.
The second way is to use the built-in compare()
function of the standard String class.
And the third way is to use the relational operators (==, !=)
.
String Comparison C++
1. strcmp() Function.
String comparison using strcmp() – C-String Method:
C-strings are arrays of type char, they are called C-strings because they were the only type of string available in the C language.
In C-strings, we need to create an array of the right size to hold the string variable, and they must terminate with a byte containing 0, which is also called the null character.
the strcmp()
function is used in C-String class to compare two strings to determine whether the strings are the same or if one comes before or after the other when arranged alphabetically. This function is specific to C-style (C-strings) strings.
#include <iostream>
#include <cstring>
Using namespace std;
int main() {
char str1[] = "tech";
char str2[] = "indetail";
int result = strcmp(str1, str2);
cout<<result<<endl;
if (result < 0) {
cout << "str1 comes before str2" << endl;
} else if (result > 0) {
cout << "str1 comes after str2" << endl;
} else {
cout << "str1 and str2 are equal" << endl;
}
return 0;
}
OUTPUT:
11
Str1 comes after str2
Code language: C++ (cpp)
The ‘strcmp()’ function returns a value less than 0 if ‘str1’ comes before ‘str2’, a value greater than 0 if ‘str1’ comes after ‘str2’, and 0 if both strings are equal.
As we can see in the OUTPUT the returned value is 11, which is greater than 0, so ‘str1’ comes after ‘str2’.
reads the file word by word and stores each word
2. Compare() function.
C++ String comparison using compare() function:
The standard C++ string class improves the C-string class in many aspects.
Now we don’t need to worry about creating an array of the right size to hold string variables.
Memory management in string class is managed automatically.
In the standard C++ string class, we have a built-in function compare()
that allows us to compare two strings.
The function returns an integer value which is used to check if the strings are equal or to determine their relative order in terms of lexicographical sorting.
Following is a C++ program that uses compare()
function to compare two strings.
#include <iostream>
#include <string>
Using namespace std;
int main() {
string str1 = "Virat";
string str2 = "Miller";
int result = str1.compare(str2);
if (result < 0) {
cout << "str1 comes before str2" << endl;
} else if (result > 0) {
cout << "str1 comes after str2" << endl;
} else {
cout << "str1 and str2 are equal" << endl;
}
return 0;
}
OUTPUT:
Str1 comes after str2.
Code language: C++ (cpp)
This program demonstrates the usage of the ‘compare’ function in C++ to compare two strings.
The logic used is the same as in the case of strcmp()
function.
We use the value returned by the compare()
function to compare the two strings.
The only difference is that in the case of compare()
function the ‘str1’ and ‘str2’ are objects of the standard C++ string class. While in the case of strcmp()
they are variables of type character arrays.
Also Read: Factorial using Recursion C++
3. Relational Operators.
String comparison using Relational Operators:
The easiest and most direct way to compare strings in C++ is by using relational operators like (==, !=, < & >)
.
C++ program to compare strings using relational operators:
// comparing string objects using relational operators
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str1 = ‘George’;
string str2 = ‘Alfred’;
if(str1 == str2)
cout<<” str1 and str2 are equal “<<endl;
else if(str1 <str2)
cout<<” str1 comes before str2 “<<endl;
else
cout<<” str1 comes after str2 “<<endl;
return 0;
OUTPUT:
str1 comes after str2
Code language: C++ (cpp)
We hope that this discussion has provided you with a clearer understanding of how to compare strings in C++.
Conclusion
In this article, you learned methods to compare strings in C++.
This included String’s strcmp()
function, the built-in compare()
function, and relational operators (==
, !=
).
Must Read: Data Structures C++
Thanks.
FAQ’s
Can I compare C-strings using the == operator?
No, the ==
operator does not work directly on C-strings. You need to use the strcmp()
function for comparing C-strings.
How does the compare() function handle case sensitivity?
By default, the compare()
function performs a case-sensitive comparison. If you want to perform a case-insensitive comparison, you can use other functions or convert the strings to a common case before comparing.
Can I compare strings of different lengths?
Yes, you can compare strings of different lengths.
The comparison is based on the individual characters up to the length of the shorter string.
The length of the strings does not affect the comparison result.