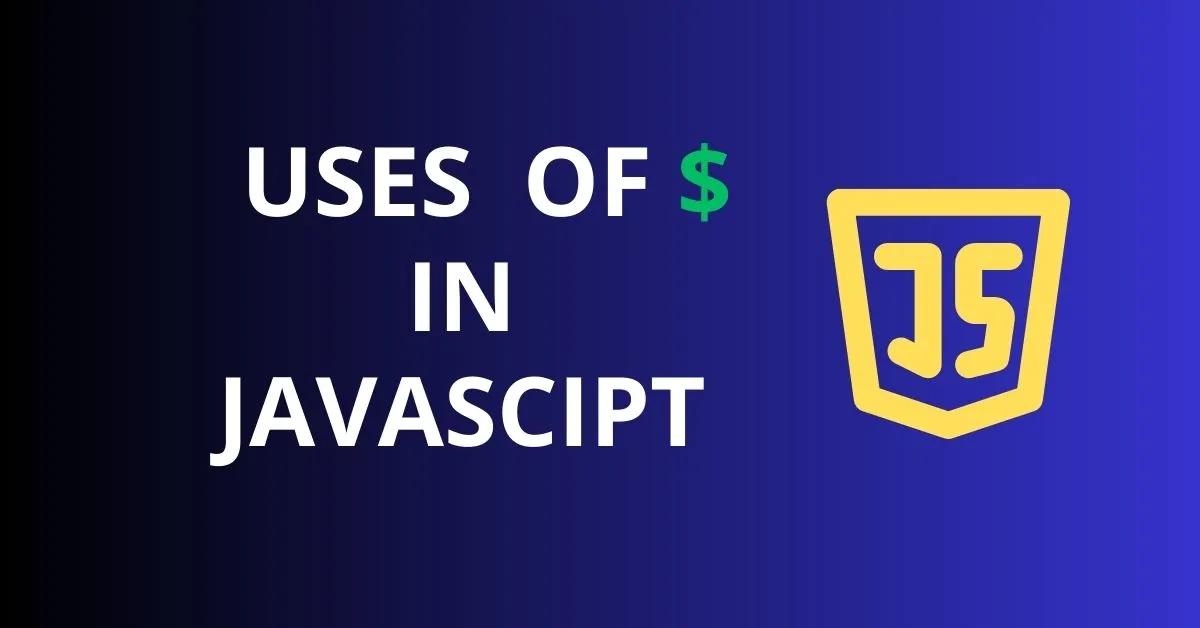
In JavaScript, the dollar sign ($) is a versatile symbol that can be used in various contexts, each with its own meaning and significance.
- Template Literals
- Variable Names
- jQuery
- Regular Expressions
- Destructuring Assignment
While the dollar sign has no inherent meaning within the JavaScript language itself, it has gained recognition through its associations with popular libraries, frameworks, and language features.
In this article, we will explore the different uses of the dollar sign in JavaScript, including its role in libraries like jQuery, template literals, variable names, regular expressions, etc.
Uses of $ in Javascript
Variable names:
The dollar sign can be used as the first character in a variable name, typically indicating a global variable.
This convention helps distinguish global variables from local ones within a program. Here’s an example:
// Declaring a global variable with a dollar sign
var $globalVar = 'Global variable value';
// Accessing the global variable
console.log($globalVar);
// Output: 'Global variable value'
Code language: PHP (php)
Regular expressions:
In regular expressions, the dollar sign is used to match a literal dollar sign character ($) within a string.
It allows for precise pattern matching and manipulation, Here’s an example:
var str = 'The price is $200';
// Matching a dollar sign character using regular expression
var match = str.match(/\$/);
console.log(match);
// Output: ['$']
Code language: JavaScript (javascript)
Template literals:
Template literals, introduced in ECMAScript 2015 (ES6), provide a convenient way to create or format strings with embedded expressions.
The dollar sign, followed by curly braces (${expression}
), plays a crucial role in variable interpolation within template literals.
var name = 'ELON';
var age = 40;
// Inserting variables into a template literal using dollar sign and curly braces
var message = `My name is ${name} and I am ${age} years old.`;
console.log(message);
// Output: 'My name is ELON and I am 40 years old.'
Code language: JavaScript (javascript)
In the example above, the variables name
and age
are enclosed within ${}
within the template literal.
During the evaluation of the template literal, the expressions inside the curly braces are executed, and their values are inserted into the resulting string.
Example 2: Function Interpolation
The dollar sign interpolation in template literals is not limited to variables alone.
It can also accommodate function calls and more advanced JavaScript expressions.
Let’s explore an example:
function capitalize(str) {
return str.charAt(0).toUpperCase() + str.slice(1);
}
const name = 'jane';
const greeting = `Hello, ${capitalize(name)}!`;
console.log(greeting);
// Output: "Hello, Jane!"
Code language: JavaScript (javascript)
In this example, the capitalize
function is invoked within the template literal by placing it inside the ${}
notation.
The function receives the name variable as an argument applies the capitalization logic, and returns the modified string.
Destructuring assignment:
The dollar sign can be used in destructuring assignments to assign the value of a property to a variable. Here’s an example:
var person = {
name: 'Alice',
age: 25,
$country: 'USA'
};
// Extracting the value of the $country property using destructuring assignment
var {$country: country} = person;
console.log(country);
// Output: 'USA'
Code language: PHP (php)
Conclusion
Although the dollar sign does not hold any inherent significance in JavaScript itself, however, it has many uses like jQuery, template literals, and regular expressions.
FAQ’s
Q1: What is the significance of the dollar sign in JavaScript variable names?
A1: The dollar sign can be used as the first character in a variable name, often indicating a global variable.
Q2: How is the dollar sign used in regular expressions?
A2: In regular expressions, the dollar sign is used to match a literal dollar sign character ($) within a string.
Q3: What is the role of the dollar sign in template literals?
A3: In template literals, the dollar sign (${expression}) facilitates variable interpolation, allowing the insertion of variable values into a string.
Q4: How is the dollar sign utilized in the destructuring assignment?
A4: The dollar sign can be used in destructuring assignments as a placeholder to extract the value of a specific property from an object and assign it to a variable.
Q5: How does the dollar sign work with spread syntax in JavaScript?
A5: With the spread syntax, the dollar sign is used to spread the contents of an iterable, such as an array or object, into another array, simplifying data manipulation and array concatenation.
Q6: Can the dollar sign have a generic placeholder role in JavaScript?
A6: Yes, apart from specific uses, the dollar sign can act as a placeholder for any character, which can be helpful for debugging or creating obfuscated code.