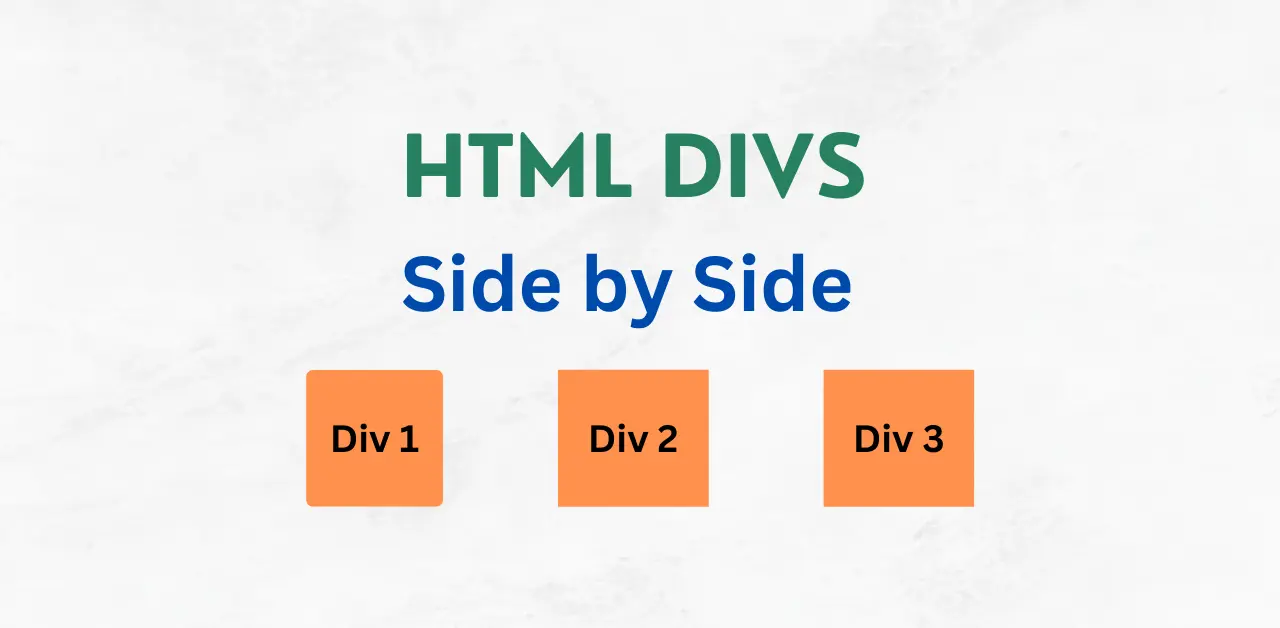
Use CSS Grid and Flexbox to display html div side by side responsive.
In modern web development, creating responsive layouts that display HTML div elements side by side is a common requirement.
CSS provides two powerful layout models, CSS Grid and Flexbox, to align HTML elements responsively.
In this article, we will explore how to use CSS Grid and Flexbox to create responsive layouts where div elements are displayed side by side.
1. Using CSS Grid
CSS Grid is a flexible and powerful layout model that allows you to create complex grid-based layouts.
Grid is ideal for arranging elements in both rows and columns, making it a great choice for displaying div
elements side by side.
Here’s an example of using CSS Grid:
<div class="grid-container">
<div class="grid-box">Box 1</div>
<div class="grid-box">Box 2</div>
<div class="grid-box">Box 3</div>
</div>
Code language: HTML, XML (xml)
CSS grid to align divs side by side
.grid-container {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(300px, 1fr));
grid-gap: 10px;
background-color: #333;
color: #fff;
}
.grid-box {
margin: 10px;
background-color: #444;
padding: 10px;
}
Code language: CSS (css)
Explanation: In the above example, the grid-container
class is set to display: grid
, which activates the CSS Grid layout for the container.
The grid-template-columns
property is used to define the number and width of the columns.
In this case, we use repeat(auto-fit, minmax(300px, 1fr))
, which instructs the browser to automatically fit as many columns as possible with a minimum width of 300 pixels (minmax(300px, 1fr)
).
The grid-gap
property adds a 10-pixel gap between the grid-box
elements.
CSS Grid achieves responsiveness by using flexible units and automatically adjusting the number of columns based on available space.
If the container becomes narrower, CSS Grid will automatically re-flow the layout to maintain an optimal display.
2. Using CSS Flexbox
Flexbox is another powerful layout model perfect for arranging elements in a single row or column.
Flexbox offers excellent flexibility and responsiveness, making it an ideal choice for displaying div
elements side by side.
Here’s an example using Flexbox:
<div class="flex-container">
<div class="flex-box">Box 1</div>
<div class="flex-box">Box 2</div>
<div class="flex-box">Box 3</div>
</div>
Code language: HTML, XML (xml)
Align divs side by side CSS Flexbox
.flex-container {
display: flex;
flex-wrap: wrap;
background-color: #333;
color: #fff;
}
.flex-box {
flex: 1 0 300px;
margin: 10px;
background-color: #444;
padding: 10px;
}
Code language: CSS (css)
Explanation: In the above example, the flex-container
class is set to display: flex
, enabling the Flexbox layout for the container.
By using flex-wrap: wrap
, the box
elements will wrap onto the next line if the available space is not sufficient.
The flex-box
class is assigned flex: 1 0 300px
, which allows each box to take up an equal amount of space within the container with a minimum width of 300 pixels.
Flexbox wraps elements onto the next line if the container becomes narrower.
This behavior ensures that the layout remains visually appealing and readable on different screen sizes.
Conclusion:
Displaying div elements side by side in a responsive manner is essential for creating modern web layouts.
You can use css grid and css flexbox to display 2 3 4 5 or many html divs side by side responsively.
CSS Grid is well-suited for complex grid-based layouts, while Flexbox excels in arranging elements in a single row or column.
By utilizing CSS Grid or Flexbox, you can create versatile and responsive designs that adapt to different screen sizes and devices.
Experiment with both approaches, consider your specific layout requirements and choose the one that best suits your needs.
FAQ’s
Q: How do I display div elements side by side using CSS Grid?
A: To display div elements side by side using CSS Grid, set the parent container to display: grid, define the columns using grid-template-columns, and adjust the width of the elements accordingly.
Q: How do I display div elements side by side using Flexbox?
A: To display div elements side by side using Flexbox, set the parent container to display: flex, adjust the flex properties of the elements, and utilize flex-wrap: wrap to wrap elements onto the next line if needed.
Q: How can I control the spacing between the side-by-side div elements?
A: You can control the spacing between the side-by-side div elements by adjusting the margins, padding, or using spacing properties like grid-gap in CSS Grid or margin in Flexbox.
Q: How can I align the side-by-side div elements vertically within the container?
A: To align the side-by-side div elements vertically within the container, you can use CSS Grid’s alignment properties such as align-items or Flexbox’s alignment properties like align-items and justify-content.
Q: Can I have different widths for the side-by-side div elements?
A: Yes, you can have different widths for the side-by-side div elements. In CSS Grid, you can define different column sizes using grid-template-columns. In Flexbox, you can adjust the flex property of each element to specify different widths.
Q: How can I make the side-by-side div elements responsive for different screen sizes?
A: To make the side-by-side div elements responsive for different screen sizes, you can utilize media queries in CSS to adjust the layout, change column widths, or modify the flex properties based on the viewport width.