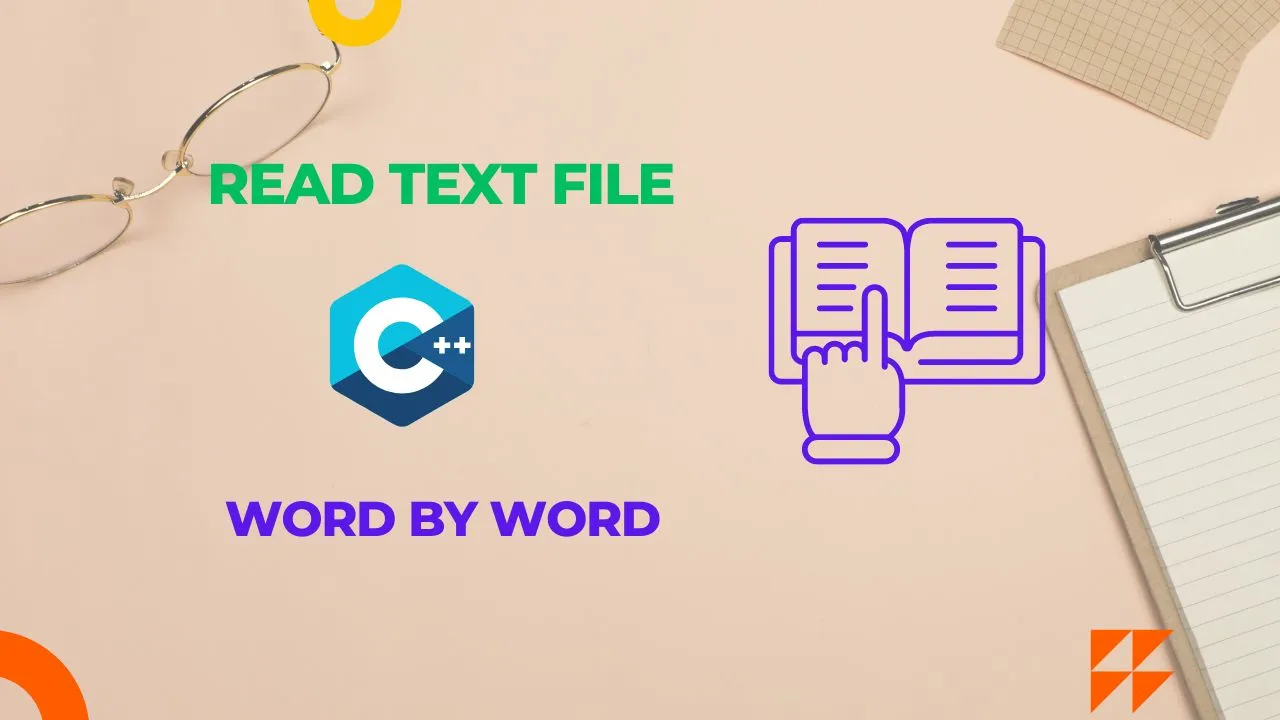
Reading a file word by word in C++ is a straightforward task that involves using the fstream
library and the extraction operator (>>
).
When working with C++, one common task is reading data from files. Reading a file word by word is a frequent requirement in various applications, such as text processing, data analysis, and more.
In this tutorial, we will explore how to read a file word by word in C++. We will cover the essential steps and provide practical examples to illustrate the process.
C++ Read File Word by Word
Prerequisites
Before we proceed, ensure you have a basic understanding of C++ programming. Familiarity with file handling concepts, such as opening, closing, reading, and writing files in C++, will be helpful.
Step 1: Including the Necessary Libraries
In C++, to work with files, we need to include the fstream
library. This library provides classes for handling file input/output operations.
To include the fstream
library in your C++ program, use the following code:
#include <iostream>
#include <fstream>
Code language: HTML, XML (xml)
The iostream
header allows us to perform input and output operations, while the fstream
header enables file stream handling.
Step 2: Opening the File
To read data from a file, we first need to open it. We can use the ifstream
class provided by the fstream
library to open an input file. Here’s how you can do it:
std::ifstream inputFile;
inputFile.open("your_file_name.txt");
Code language: CSS (css)
Replace "your_file_name.txt"
with the name of the file, you want to read. Make sure the file is in the same directory as your C++ program, or provide the correct path to the file.
Step 3: Reading the File Word by Word
Once the file is open, we can read its content word by word. In C++, we can use the extraction operator (>>
) to read words from the file.
The extraction operator reads a sequence of characters until it encounters whitespace (space, tab, newline, etc.).
It then stores the extracted word in a variable. Here’s how you can read words from the file:
std::string word;
while (inputFile >> word) {
// Process the word here
}
Code language: PHP (php)
In this code snippet, we use a while
loop to keep reading words from the file until there are no more words to read. The loop will terminate when it reaches the end of the file.
Step 4: Processing the Words
Inside the while
loop, you can process each word as per your application’s requirements.
Let’s explore some real-life examples and use cases to better understand the practical applications of reading a file word by word in C++:
- Text Analysis: Word-by-word reading is essential for performing text analysis tasks like counting the occurrences of specific words, finding the most frequent words, or calculating the average word length in a document.
- Spell Checking: By reading words from a reference dictionary file and comparing them to the words in the input text, you can implement a simple spell-checking mechanism, flagging misspelled words for correction.
- Language Translation: When building language translation tools, reading words from a source file helps you convert sentences word by word, making the translation process more efficient.
- Natural Language Processing (NLP): In NLP applications, word-by-word reading is used to preprocess text data, tokenize sentences, and identify parts of speech, which forms the foundation for various language understanding tasks.
- Keyword Extraction: For search engines or content analysis tools, reading words from articles or documents is necessary for extracting important keywords that represent the document’s content.
Step 5: Handling Errors
File operations can sometimes fail, such as when the file does not exist or cannot be opened.
It is essential to handle such scenarios gracefully. To check if the file was opened successfully, you can use an if
statement:
if (!inputFile) {
std::cerr << "Error opening the file." << std::endl;
return 1;
}
Code language: PHP (php)
The ifstream
class has an implicit conversion to bool
, which evaluates to false
if the file opening fails.
The error message will be displayed on the standard error stream (cerr
), and the program will return a non-zero value (1) to indicate an error.
Step 6: Closing the File
Once you are done reading the file, it is essential to close it properly. Closing the file releases the resources used by the file stream. To close the file, use the following code:
inputFile.close();
Code language: CSS (css)
By closing the file, you ensure that the data is correctly written and avoid potential data loss or corruption.
C++ Program Read File Word by Word
Let’s put it all together in a complete example:
#include <iostream>
#include <fstream>
int main() {
std::ifstream inputFile;
inputFile.open("example.txt");
if (!inputFile) {
std::cerr << "Error opening the file." << std::endl;
return 1;
}
std::string word;
while (inputFile >> word) {
std::cout << word << " ";
}
inputFile.close();
return 0;
}
Code language: PHP (php)
In this example program, assuming you have a file named “example.txt” with the content “Hello World,” the program will output “Hello World” to the console.
Conclusion
In this tutorial, we learned how to read a file word by word in C++.
We covered the necessary steps, including the fstream
library, opening the file, reading the words, processing them, and finally, closing the file.
We also explored some real-life examples and use cases to demonstrate the practical applications of reading a file word by word.
Faq’s
Q1: Can I use C++ to read a file word by word?
A1: Yes, you can use C++ to read a file word by word by using the fstream
library and the extraction operator (>>
).
Q2: How do I include the fstream
library in my C++ program?
A2: To include the fstream
library, use the following line of code at the beginning of your C++ program: #include <fstream>
.
Q3: Are there other ways to read a file in C++ besides word-by-word reading?
A3: Yes, besides word-by-word reading, you can read a file character by character or line by line using different techniques in C++.
Q4: How can I count the total number of words in a file using C++?
A4: You can achieve this by incrementing a counter variable inside the while
loop that reads words from the file. The counter will represent the total number of words in the file.