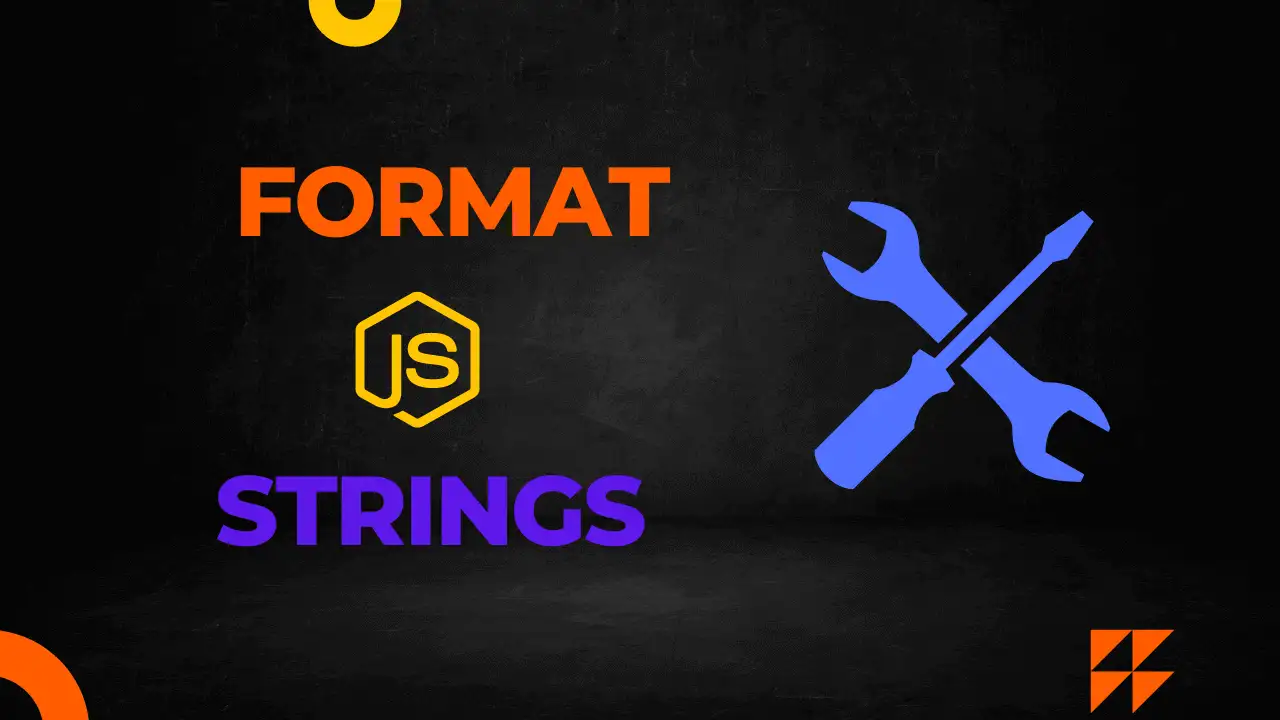
Welcome to our quick and concise guide on JavaScript string format.
3 simple ways to format strings in JavaScript.
- string concatenation (+)
- backticks (` `) (Recommended)
- Custom Function
In this JS tutorial, you will learn string formatting in JavaScript or how to format strings in javascript.
All you need to do is to join two strings or to join a string and a number by the following 3 methods.
Javascript String Formating Methods
1. String Concatenation
In JavaScript, the + symbol is treated as a concatenation operator that joins two strings or variables as a string.
This is the most simple and basic way to join or concatenate two strings in javascript. For Example,
console.log('Java' + 'Script');
// output JavaScript
console.log(‘JavaScript’ + 6)
// output JavaScript6
Code language: JavaScript (javascript)
Also, in the following code let’s join two string variables.
const language = "JS";
const type = "Tutorial";
<em>let</em> output = "Hi this is a " + language + " string " + type;
console.log(output);
// Hi this is a JS string Tutorial
Code language: JavaScript (javascript)
In this example, the value of two const strings is concatenated into the output string.
Example 2:
let name = "Alice";
let age = 25;
let output = "Hi! The age of " + name + "is" + age + "years old";
// Hi! The age of Alice is 25 years old.
Code language: JavaScript (javascript)
This string formatting method is easy but it should not be used if you have to calculate values during formatting.
Note:- Do not use this in mathematical calculations.
For example, the following number expression produces the wrong output.
console.log("The sum of 4 + 3 is " + 4 + 3);
// "Sum of 4 + 3 is 43"
Code language: JavaScript (javascript)
As you can see, in the example above adding the numbers 4 + 3 gives an output of 43 instead of 7.
Because 4, and 3 are considered strings, not numbers, to properly output this type of expression you need to wrap the expression in curly braces as below.
console.log("The sum of 4 + 3 is " + (4 + 3));
// Output The sum of 4 + 3 is 7
Code language: JavaScript (javascript)
2. Template Literals
The template literals were added to the ES6, and this is the recommended way to insert variables and expressions into the strings.
Template literals can be used to create multi-line strings and insert variables and number expressions into the strings.
Instead of single quotes or double quotes, we use backticks to wrap the whole string, inside these backticks we can use strings and variables.
In a template string, inside the backticks the normal text is written as it is however, to insert variables or expressions a $ sign is appended, and the actual expression is placed inside the curly braces { }.
let name = "Bob";
let role = "Wordpress Developer";
let output = `Hello! My name is ${name} and I'm a ${role}`;
console.log(output);
// Hello! My name is Bob and I'm a WordPress Developer
Code language: JavaScript (javascript)
Here, ${name}
and ${role}
acts as a placeholder and is replaced with the actual value of the variable.
Also, to insert numbers inside strings, the same method is followed starting with the dollar sign $ and placing the numbers inside the curly braces {}.
console.log(`The sum of 4 + 3 is ${4 + 3}`);
// The sum of 4 + 3 i s 7
Code language: JavaScript (javascript)
You can also pass a function in the template literals similarly to variables.
// function definition
function student(name, id) {
return `${name} ${id}`;
}
console.log(`${fullName("Alice", 07)}`);
// Output Alice07
Code language: JavaScript (javascript)
Also Read: Round Numbers in Javascript
3. Custom Function
You can use custom functions to format strings in JavaScript because in JS there is no default function for that.
In this method, you have to use regular expressions to search and replace the template numbers with the arguments passed into the function.
if (!String.prototype.format) {
String.prototype.format = function () {
var args = arguments;
// if args , then replace match with args
return this.replace(/{(\d+)}/g, function (match, number) {
// check if the argument is present
return typeof args[number] != "undefined" ? args[number] : match;
});
};
}
Code language: JavaScript (javascript)
The format()
the method above searches the placeholders like {0}
and {1}
and replaces these with the arguments you pass into the method.
let name = "Alice"
let role = "Wordpress Developer"
let aString = "My name is {0}, I'm a {1}".format(name, role);
console.log(aString);
// My name is Alice, I'm a WordPress Developer
Code language: JavaScript (javascript)
See Also: Table of Contents In JS
Conclusion:
In general, the recommended way for formatting strings in javascript is using the template strings (backticks) and for the very basic string formatting use the concatenation (+) method.
Now that you’ve mastered JavaScript string formatting, you’re well-equipped to handle and manipulate strings like a pro.
By applying these techniques in your projects, you’ll create more readable and structured code. Keep practicing, and soon you’ll be crafting impressive string manipulations effortlessly.
See Also: setInterval vs setTimeout JS
Faq’s
Q: How do I concatenate strings in JavaScript?
A: To concatenate strings, you can simply use the +
operator. For example, var firstName = "John"; var lastName = "Doe"; var fullName = firstName + " " + lastName;
will result in “John Doe”.
Q: What is string interpolation in JavaScript?
A: String interpolation allows you to embed expressions within a string using template literals (backticks). For instance, ${}
syntax helps you insert variables or expressions directly into the string. Example: const age = 30; const message = `I am ${age} years old.`;
Q: How can I capitalize the first letter of a string in JavaScript?
A: You can capitalize the first letter of a string using various methods. One simple way is: const str = "hello"; const capitalizedStr = str.charAt(0).toUpperCase() + str.slice(1);
, which results in “Hello”.
Q: How do I remove leading and trailing whitespaces from a string?
A: JavaScript provides trim()
a method for this purpose. For instance, const text = " Hello, World! "; const trimmedText = text.trim();
will result in “Hello, World!” with no extra spaces.
Q: Can I format numbers inside a string in JavaScript?
A: Yes, you can use the toLocaleString()
method to format numbers with commas as thousands of separators based on the user’s locale. Example: const num = 1234567.89; const formattedNum = num.toLocaleString();
, which returns “1,234,567.89”.
Q: How can I repeat a string a certain number of times in JavaScript?
A: You can use the repeat()
method to repeat a string. For example, "Hello,".repeat(3)
will produce “Hello,Hello,Hello,”.
Q: How can I replace specific text in a string with JavaScript?
A: You can use the replace()
method, which takes a regular expression or a substring to be replaced and the new text.
For instance, const sentence = "I love apples."; const newSentence = sentence.replace("apples", "bananas");
will give “I love bananas.”
Q: Can I format dates as strings in JavaScript?
A: Yes, you can format dates using the toLocaleDateString()
method. Example: const date = new Date(); const formattedDate = date.toLocaleDateString();
, which formats the date based on the user’s locale.
Q: How do I convert a string to lowercase or uppercase in JavaScript?
A: You can use the toLowerCase()
or toUpperCase()
methods, respectively. For example, "Hello".toLowerCase()
returns “hello”, and "World".toUpperCase()
returns “WORLD”.