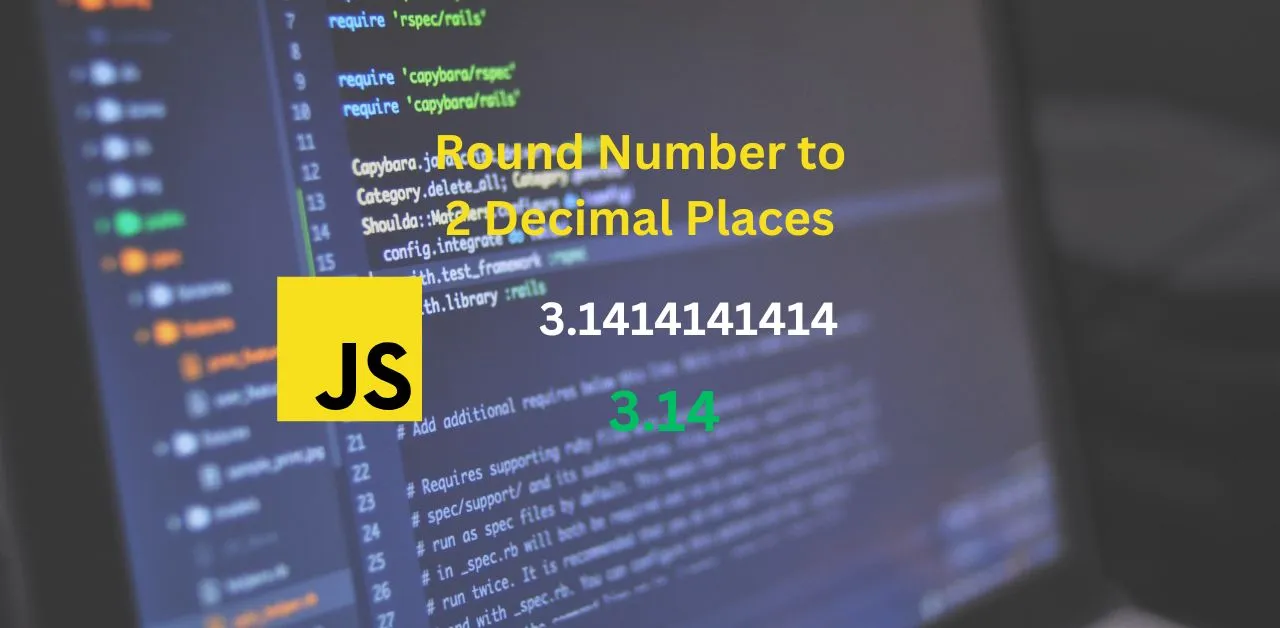
The 2 simple methods to round numbers to 2 3 4 decimal places in JavaScript are toFixed() and Math.random() in built functions.
When working with numbers in JavaScript, you may often need to round them to a specific number of decimal places.
In this article, we will explore how to round numbers to 2, 3, and 4 decimal places using JavaScript.
Method 1: Using toFixed() Method
JavaScript provides the toFixed()
method, which allows you to round a number to a specified number of decimal places and obtain a string representation of the rounded value.
The syntax for using toFixed()
is as follows:
number.toFixed(decimalPlaces);
Code language: CSS (css)
Here, number
is the original number you want to round, and decimalPlaces
is the desired number of decimal places you want to round to.
Let’s look at an example to round a number to 2 decimal places:
var number = 3.14159;
var roundedNumber = number.toFixed(2); // "3.14"
Code language: JavaScript (javascript)
In the above example, number.toFixed(2)
returns the string "3.14"
, which represents the rounded value of 3.14159
to 2 decimal places.
Similarly, you can round a number to 3 or 4 decimal places by adjusting the decimalPlaces
argument accordingly.
Here are examples for rounding to 3 and 4 decimal places:
var number = 3.14159;
var roundedNumber3 = number.toFixed(3); // "3.142"
var roundedNumber4 = number.toFixed(4); // "3.1416"
Code language: JavaScript (javascript)
In the above examples, number.toFixed(3)
returns the string "3.142"
, rounding 3.14159
to 3 decimal places, and number.toFixed(4)
returns the string "3.1416"
, rounding it to 4 decimal places.
It’s important to note that the toFixed()
method returns a string representation of the rounded number, not a numeric value.
If you need to perform further calculations with the rounded number, you may need to convert it back to a number using parseFloat()
or Number()
functions.
var number = 3.14159;
var roundedNumber2 = parseFloat(number.toFixed(3));
// 3.14 is now number
Code language: JavaScript (javascript)
Method 2: Using Math.round() Function
If you prefer to round a number to a specific number of decimal places and obtain a numeric value instead of a string, you can use the Math.round()
function.
The Math.round()
function rounds a number to the nearest integer.
By multiplying and dividing the number by the appropriate factor, we can achieve rounding to a specific number of decimal places.
Let’s see how we can round a number to 2 decimal places using Math.round()
:
var number = 3.14159;
var roundedNumber = Math.round(number * 100) / 100;
// 3.14 output
Code language: JavaScript (javascript)
In the above example, we multiply the number by 100
to shift the decimal places two positions to the right. We then use Math.round()
to round it to the nearest integer.
Finally, we divide the result by 100
to restore the original scale, giving us the rounded value of 3.14159
to 2 decimal places.
Similarly, you can round a number to 3 or 4 decimal places by adjusting the multiplication and division factors accordingly:
var number = 3.14159;
// Rounding to 3 decimal places
var roundedNumberThree = Math.round(number * 1000) / 1000;
// 3.142 output
// Rounding to 4 decimal places
var roundedNumberFour = Math.round(number * 10000) / 10000;
// 3.1416 output
Code language: JavaScript (javascript)
In the above examples, Math.round(number * 1000) / 1000
rounds 3.14159
to 3 decimal places, and Math.round(number * 10000) / 10000
rounds it to 4 decimal places.
Remember, when using Math.round()
for rounding to a specific number of decimal places, the multiplication and division factors should be powers of 10 to shift the decimal places accordingly.
Conclusion
Rounding numbers to a specific number of decimal places is a common requirement in JavaScript programming.
In this article, we explored two methods to round numbers in javascript to 2 3 4 decimal places according to our needs.
By using the toFixed()
method and the Math.round()
function.
Thanks!
FAQ’s
Q: How can I round a number to the nearest integer in JavaScript?
A: You can use the Math.round()
function to round a number to the nearest integer.
Q: How can I get the absolute value of a number in JavaScript?
A: You can use the Math.abs()
function to get the absolute value of a number.
Q: How can I limit a number within a specific range in JavaScript?
A: You can use Math.min()
and Math.max()
functions to limit a number within a specific range. For example, Math.min(maxValue, Math.max(minValue, number))
.
Q: How can I generate a random number with a decimal in JavaScript?
A: You can use Math.random()
function and multiply it by a desired factor to generate a random number with a decimal.
For example, to generate a random number between 0 and 1.5: Math.random() * 1.5
.
Q: How to round to 2 decimals in JavaScript?
A: You can use the toFixed()
method or multiply and divide the number by a factor of 10 to round to 2 decimal places in JavaScript.
Q: How to reduce decimal places in JavaScript?
A: You can use the toFixed()
method with a smaller argument to round the number to the desired decimal places.
or multiply the number by an appropriate factor, round it, and then divide by the same factor to reduce the decimal places.